用链表写个图书管理程序
时间: 2024-03-13 15:21:07 浏览: 22
好的,我可以帮您写一个图书管理程序。以下是一个示例代码,使用单向链表来实现图书的添加、删除和查询:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct book {
char title[100];
char author[50];
int year;
struct book* next;
} Book;
Book* create_book(char* title, char* author, int year) {
Book* new_book = (Book*)malloc(sizeof(Book));
strcpy(new_book->title, title);
strcpy(new_book->author, author);
new_book->year = year;
new_book->next = NULL;
return new_book;
}
void add_book(Book** head, char* title, char* author, int year) {
Book* new_book = create_book(title, author, year);
if (*head == NULL) {
*head = new_book;
return;
}
Book* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = new_book;
}
void delete_book(Book** head, char* title) {
Book* current = *head;
Book* previous = NULL;
while (current != NULL) {
if (strcmp(current->title, title) == 0) {
if (previous == NULL) {
*head = current->next;
} else {
previous->next = current->next;
}
free(current);
return;
}
previous = current;
current = current->next;
}
}
void search_books(Book* head, char* author) {
Book* current = head;
while (current != NULL) {
if (strcmp(current->author, author) == 0) {
printf("%s (%d) by %s\n", current->title, current->year, current->author);
}
current = current->next;
}
}
void print_books(Book* head) {
Book* current = head;
while (current != NULL) {
printf("%s (%d) by %s\n", current->title, current->year, current->author);
current = current->next;
}
}
int main() {
Book* library = NULL;
add_book(&library, "The Catcher in the Rye", "J.D. Salinger", 1951);
add_book(&library, "To Kill a Mockingbird", "Harper Lee", 1960);
add_book(&library, "1984", "George Orwell", 1949);
add_book(&library, "Pride and Prejudice", "Jane Austen", 1813);
printf("All books:\n");
print_books(library);
printf("\nDeleting '1984'...\n");
delete_book(&library, "1984");
printf("\nBooks by J.D. Salinger:\n");
search_books(library, "J.D. Salinger");
return 0;
}
```
这个程序可以添加、删除和查询图书,您可以根据需要进行修改。请问还有其他问题需要我回答吗?
相关推荐
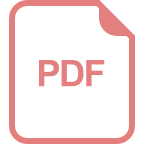
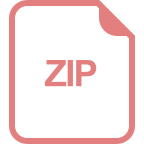














