jsonArray.stream().filter(json->json)获取值
时间: 2023-08-12 17:31:10 浏览: 257
这段代码是有问题的,因为在 filter() 方法中应该传入一个 Lambda 表达式,这个 Lambda 表达式的参数类型应该与 jsonArray 中元素的类型相同,同时返回值应该是一个 boolean 类型的值,用于过滤 jsonArray 中不符合条件的元素。而这里传入的 Lambda 表达式中,参数名为 json,但是没有定义参数的类型,也没有定义返回值,因此是无法编译通过的。
如果您想要筛选出 jsonArray 中某个属性值为 true 的元素,可以使用类似如下的代码:
```
jsonArray.stream()
.filter(json -> json.getBoolean("propertyName"))
.forEach(json -> {
// 处理符合条件的元素
});
```
这里的 "propertyName" 是指 jsonArray 中每个元素中需要筛选的属性名。如果您需要对其他数据类型的 jsonArray 进行过滤,可以根据实际情况修改 Lambda 表达式的参数类型和返回值类型。
相关问题
JSONArray jsonArray = JSONObject.parseArray(commodityDetailsList); jsonArray.stream().filter() filter怎么获取值
在使用 `filter()` 过滤 `JSONArray` 中的元素时,可以使用 `JSONObject` 中提供的方法来获取每个元素中的属性值,例如:
```
jsonArray.stream()
.filter(json -> json.getInteger("id") == 1) // 过滤 id 值为 1 的元素
.forEach(json -> {
// 处理符合条件的元素
System.out.println(json.getString("name")); // 输出符合条件元素的 name 属性值
});
```
在这个例子中,我们通过 `json.getInteger("id") == 1` 的方式来过滤 `id` 属性值为 1 的元素,然后在 `forEach()` 中处理符合条件的元素,这里只是简单地输出了元素的 `name` 属性值。
您可以根据实际情况修改 Lambda 表达式中的过滤条件和处理逻辑。
List<SchemaRowRule> userRowRuleList = schemaRowRuleList.stream() .filter(schemaRowRuleEo -> { List<String> userList = JSONArray.parseArray(schemaRowRuleEo.getRowPermissionUserList(), String.class); List<String> orgList = JSONArray.parseArray(schemaRowRuleEo.getRowPermissionOrgList(), String.class); return userList.contains(userUid) || orgList.contains(orgCode); }) .map(schemaRowRuleEo -> { SchemaRowRule schemaRowRule = SchemaRowRule.builder().build(); BeanUtils.copyProperties(schemaRowRuleEo, schemaRowRule); return schemaRowRule; }) .collect(Collectors.toList()); List<SchemaColumnRule> userColumnRuleList = schemaColumnRuleList.stream() .filter(rule -> { if (StringUtils.isNotEmpty(rule.getColumnPermissionUserList())) { List<String> userList = JSONArray.parseArray(rule.getColumnPermissionUserList(), String.class); return userList.contains(userUid); } else if (StringUtils.isNotEmpty(rule.getColumnPermissionOrgList())) { List<String> orgList = JSONArray.parseArray(rule.getColumnPermissionOrgList(), String.class); return orgList.contains(orgCode); } return false; }) .map(rule -> { SchemaColumnRule columnRule = new SchemaColumnRule(); BeanUtils.copyProperties(rule, columnRule); return columnRule; }) .collect(Collectors.toList());优化代码
可以考虑使用方法引用来简化Lambda表达式,同时避免多次解析JSON字符串,代码如下:
```
List<SchemaRowRule> userRowRuleList = schemaRowRuleList.stream()
.filter(schemaRowRuleEo -> {
List<String> userList = JSONArray.parseArray(schemaRowRuleEo.getRowPermissionUserList(), String.class);
List<String> orgList = JSONArray.parseArray(schemaRowRuleEo.getRowPermissionOrgList(), String.class);
return userList.contains(userUid) || orgList.contains(orgCode);
})
.map(schemaRowRuleEo -> {
SchemaRowRule schemaRowRule = new SchemaRowRule();
BeanUtils.copyProperties(schemaRowRuleEo, schemaRowRule);
return schemaRowRule;
})
.collect(Collectors.toList());
List<SchemaColumnRule> userColumnRuleList = schemaColumnRuleList.stream()
.filter(rule -> {
List<String> userList = Optional.ofNullable(rule.getColumnPermissionUserList())
.map(jsonArray -> JSONArray.parseArray(jsonArray, String.class))
.orElse(Collections.emptyList());
List<String> orgList = Optional.ofNullable(rule.getColumnPermissionOrgList())
.map(jsonArray -> JSONArray.parseArray(jsonArray, String.class))
.orElse(Collections.emptyList());
return userList.contains(userUid) || orgList.contains(orgCode);
})
.map(BeanUtils::instantiate)
.collect(Collectors.toList());
```
其中,使用了`Optional`来避免`null`值的判断和处理,使用`BeanUtils::instantiate`方法引用来创建对象并复制属性。
阅读全文
相关推荐
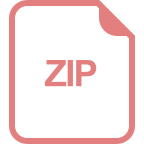


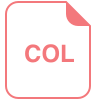
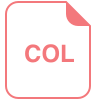
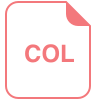
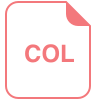
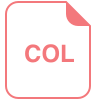
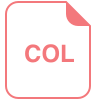
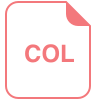
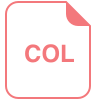



