Fluent udf 读写文件并行化
时间: 2023-09-07 13:17:16 浏览: 135
在 Fluent 中使用 UDF 读写文件并行化可以通过以下步骤实现:
1. 在 UDF 中使用 MPI 库,将读写文件的代码封装在 MPI 进程中。
2. 使用 Fluent 提供的 parallel I/O API,将读写文件的操作分配到不同的 MPI 进程中,实现并行读写。
具体实现步骤如下:
1. 初始化 MPI 环境,获取当前进程号和总进程数。
```
#include "mpi.h"
MPI_Init(&argc, &argv);
int rank, size;
MPI_Comm_rank(MPI_COMM_WORLD, &rank);
MPI_Comm_size(MPI_COMM_WORLD, &size);
```
2. 使用 MPI 进程将读写文件的操作封装起来。例如,读取文件并计算每行的平均值:
```
double sum = 0;
int count = 0;
if (rank == 0) {
FILE *fp = fopen("input.txt", "r");
char line[100];
while (fgets(line, sizeof(line), fp) != NULL) {
double num = atof(line);
sum += num;
count++;
}
fclose(fp);
}
double avg = 0;
MPI_Reduce(&sum, &avg, 1, MPI_DOUBLE, MPI_SUM, 0, MPI_COMM_WORLD);
if (rank == 0) {
avg /= count;
printf("Average: %f\n", avg);
}
```
3. 使用 Fluent 提供的 parallel I/O API,将读写文件操作分配到不同的 MPI 进程中。例如,使用 `rpwrite` 函数写入文件:
```
rpwrite("output.txt", "text", strlen("text"), 0, RP_FILE_ASCII);
```
在这个例子中,`rpwrite` 函数将 "text" 写入名为 "output.txt" 的文件中,第四个参数是写入的偏移量,可以根据需要指定。在 Fluent 中,每个进程都有自己的文件描述符,可以同时写入同一个文件,Fluent 会自动处理并发写入的问题。
4. 在 UDF 中使用 `rpgetsize` 函数获取文件大小,并使用 `rpread` 函数读取文件内容。例如:
```
int size = rpgetsize("input.txt");
char *buffer = malloc(size + 1);
memset(buffer, 0, size + 1);
rpread("input.txt", buffer, size, 0, RP_FILE_ASCII);
```
在这个例子中,`rpgetsize` 函数获取 "input.txt" 文件的大小,`rpread` 函数将文件内容读取到 `buffer` 中。
需要注意的是,使用 parallel I/O API 读写文件时,文件路径需要使用绝对路径,否则会出现文件找不到的错误。另外,在使用 parallel I/O API 时,不要使用 C 库中的标准 I/O 函数(如 fopen、fread 等),否则会导致文件锁定和性能下降。
相关推荐
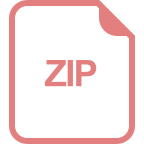
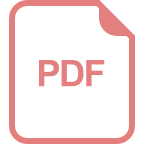
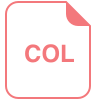













