移动机器人点到点控制代码
时间: 2023-09-14 21:12:11 浏览: 61
移动机器人点到点控制的代码可以根据不同的机器人和运动控制器而异,但是一般都包括以下几个步骤:
1. 读取当前机器人的位置和目标位置;
2. 计算机器人需要移动的距离和方向;
3. 发送运动控制指令,使机器人向目标位置移动;
4. 循环执行步骤1-3,直到机器人到达目标位置。
以下是一个简单的示例代码,用于控制一个基于ROS的移动机器人向指定的目标位置移动(假设机器人已经连接到ROS系统,并且已经安装了必要的软件包):
```python
#!/usr/bin/env python
import rospy
from geometry_msgs.msg import Twist
from nav_msgs.msg import Odometry
# 定义当前机器人的位置和目标位置
current_pos = Odometry()
target_pos = Odometry()
# 订阅当前机器人的位置信息
def odom_callback(data):
global current_pos
current_pos = data
rospy.init_node('move_robot', anonymous=True)
# 订阅机器人的odom话题,获取机器人当前的位置信息
rospy.Subscriber('/odom', Odometry, odom_callback)
# 发布机器人的运动控制指令
pub = rospy.Publisher('/cmd_vel', Twist, queue_size=10)
# 设置机器人的目标位置
target_pos.pose.pose.position.x = 1.0
target_pos.pose.pose.position.y = 1.0
rate = rospy.Rate(10) # 10hz
while not rospy.is_shutdown():
# 计算机器人需要移动的距离和方向
dx = target_pos.pose.pose.position.x - current_pos.pose.pose.position.x
dy = target_pos.pose.pose.position.y - current_pos.pose.pose.position.y
distance = math.sqrt(dx*dx + dy*dy)
angle = math.atan2(dy, dx)
# 发送控制指令,使机器人向目标位置移动
twist = Twist()
twist.linear.x = 0.5 * distance # 假设机器人的最大线速度为0.5m/s
twist.angular.z = 1.0 * angle # 假设机器人的最大角速度为1.0rad/s
pub.publish(twist)
# 到达目标位置后停止移动
if distance < 0.1:
twist.linear.x = 0.0
twist.angular.z = 0.0
pub.publish(twist)
break
rate.sleep()
```
这段代码中,我们使用了ROS的通信机制,订阅了机器人的odom话题,获取机器人当前的位置信息;同时,我们也发布了机器人的运动控制指令,使机器人向目标位置移动。在每次循环中,我们都计算机器人需要移动的距离和方向,然后根据机器人的最大线速度和角速度,计算出机器人的运动控制指令并发送给机器人。当机器人到达目标位置后,我们停止机器人的运动,并退出程序。
相关推荐
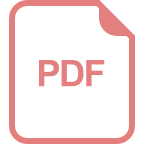
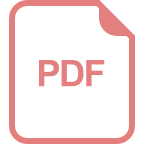
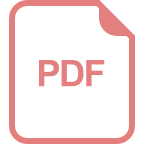














