差动运动学模型机器人从A点移动到B点的C++语言代码案例
时间: 2023-09-09 20:05:20 浏览: 83
### 回答1:
差动运动学模型机器人从A点移动到B点的C语言代码案例可以参考下面的示例:#include <stdio.h>int main(){
int A_x = 0;
int A_y = 0;
int B_x = 10;
int B_y = 10;
int x = A_x;
int y = A_y; while (x < B_x || y < B_y){
if (x < B_x){
x++;
}
if (y < B_y){
y++;
}
printf("Robot at x: %d, y: %d\n", x, y);
} return 0;
}
### 回答2:
要用C语言编写差动运动学模型机器人从点A移动到点B的代码案例,我们可以使用简单的算法来控制机器人的移动。
首先,我们需要定义机器人的初始位置、目标位置以及机器人的速度。假设机器人在二维平面上移动,我们可以用(x, y)的坐标表示机器人的位置。
接下来,我们可以利用循环来实现机器人的移动。每次循环,我们计算机器人与目标位置之间的距离,并根据差动运动学模型来调整机器人的速度和方向。
具体的代码如下:
```c
#include <stdio.h>
#include <math.h>
#define TARGET_THRESHOLD 0.1 // 目标位置阈值
#define SPEED 0.1 // 机器人移动速度
int main() {
double current_x = /* A点的x坐标 */;
double current_y = /* A点的y坐标 */;
double target_x = /* B点的x坐标 */;
double target_y = /* B点的y坐标 */;
// 计算机器人与目标位置之间的距离
double distance = sqrt(pow(target_x - current_x, 2) + pow(target_y - current_y, 2));
while (distance > TARGET_THRESHOLD) {
// 计算机器人的角度和速度
double angle = atan2(target_y - current_y, target_x - current_x);
double velocity_x = SPEED * cos(angle);
double velocity_y = SPEED * sin(angle);
// 更新机器人的位置
current_x += velocity_x;
current_y += velocity_y;
// 重新计算机器人与目标位置之间的距离
distance = sqrt(pow(target_x - current_x, 2) + pow(target_y - current_y, 2));
// 打印机器人的当前位置
printf("当前位置:(%lf, %lf)\n", current_x, current_y);
}
// 到达目标位置
printf("到达目标位置!\n");
return 0;
}
```
在代码中,我们先定义机器人的初始位置和目标位置,然后使用循环来计算机器人与目标位置之间的距离,并根据差动运动学模型来调整机器人的速度和方向,直到机器人到达目标位置。
此代码案例只是一个简单示例,实际的机器人控制可能需要更复杂的算法和传感器输入。
相关推荐
















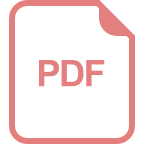