将两份代码综合为一份代码
时间: 2023-07-03 12:27:23 浏览: 38
好的,让我们将之前的两份代码综合为一份代码,这样就可以实现类student和对话机器人的功能了。以下是示例代码:
```c++
#include <iostream>
#include <string>
#include <vector>
#include <ctime>
#include <cstdlib>
using namespace std;
// 类student定义
class student {
public:
string name;
int age;
float score;
// 默认构造函数
student() {
name = "";
age = 0;
score = 0.0f;
}
// 构造函数
student(string n, int a, float s) {
name = n;
age = a;
score = s;
}
void display() {
cout << "Name: " << name << "\nAge: " << age << "\nScore: " << score << endl;
}
};
// 对话机器人定义
class DialogAgent {
private:
vector<string> responses;
public:
// 构造函数
DialogAgent() {
responses.push_back("Hello there!");
responses.push_back("How are you doing today?");
responses.push_back("What can I do for you?");
responses.push_back("I'm sorry, I don't understand.");
responses.push_back("Can you please elaborate?");
responses.push_back("That's interesting. Tell me more.");
responses.push_back("I'm glad to hear that!");
responses.push_back("What do you think about that?");
responses.push_back("I'm sorry, I cannot help you with that.");
}
// 对话函数
string talk(string input) {
srand(time(NULL)); // 用系统时间初始化随机数生成器
int index = rand() % responses.size(); // 随机选择一个回复
return responses[index];
}
};
// 主函数
int main() {
student s1; // 调用默认构造函数
student s2("Tom", 18, 90.5);
s1.display();
s2.display();
DialogAgent bot;
string input;
cout << "Say something to the bot: ";
getline(cin, input);
while (input != "bye") {
string response = bot.talk(input);
cout << "Bot: " << response << endl;
cout << "You: ";
getline(cin, input);
}
cout << "Bot: Goodbye!" << endl;
return 0;
}
```
在上述代码中,我们将类student和对话机器人综合到了一起。在主函数中,我们首先创建了两个学生对象,一个使用默认构造函数进行初始化,另一个使用自定义构造函数进行初始化。然后,我们创建了一个对话机器人对象,并在while循环中不断与用户进行对话,直到用户输入“bye”为止。每次用户输入后,机器人都会随机选择一个回复作为响应。最后,机器人说“Goodbye!”并结束程序。
相关推荐
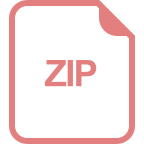
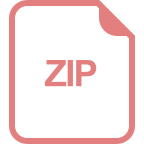
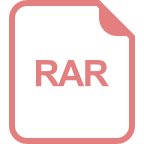














