使用js将下列的工资表保存到变量salary里,工时表保存到hours里。 1.网页上显示一个姓名下拉列表,选择对应的姓名,下面动态显示工资表和工时表,及对应的工资和工时小计。 2.统计员工时薪最高的2位员工姓名、总薪资、总工时和平均时薪,并将结果以美观大方样式输出到HTML页面里。
时间: 2024-02-18 10:04:14 浏览: 25
好的,我会回答你的问题,但是请注意,我是一个语言模型,无法实际执行代码,我只能提供一些思路和代码示例。
首先,需要将工资表和工时表保存到变量里。可以使用 JavaScript 的数组和对象来保存数据。示例代码如下:
```javascript
// 工资表
var salary = [
{ name: '张三', salary: 5000 },
{ name: '李四', salary: 6000 },
{ name: '王五', salary: 7000 }
];
// 工时表
var hours = [
{ name: '张三', hours: 160 },
{ name: '李四', hours: 180 },
{ name: '王五', hours: 200 }
];
```
接着,需要在网页上创建一个下拉列表,用于选择对应的姓名。可以使用 HTML 的 `<select>` 元素和 JavaScript 的 DOM 操作来实现。示例代码如下:
```html
<select id="name">
<option value="张三">张三</option>
<option value="李四">李四</option>
<option value="王五">王五</option>
</select>
<div id="salaryTable"></div>
<div id="hoursTable"></div>
<div id="subtotal"></div>
```
```javascript
var select = document.getElementById('name');
select.addEventListener('change', function() {
var name = select.value;
// 根据姓名查找工资和工时
var s = salary.find(function(item) { return item.name === name; });
var h = hours.find(function(item) { return item.name === name; });
// 显示工资表和工时表
document.getElementById('salaryTable').innerText = '工资:' + s.salary;
document.getElementById('hoursTable').innerText = '工时:' + h.hours;
// 计算小计
var subtotal = s.salary * h.hours;
document.getElementById('subtotal').innerText = '小计:' + subtotal;
});
```
最后,需要统计员工时薪最高的2位员工的信息,并将结果以美观大方样式输出到 HTML 页面里。可以使用 JavaScript 的数组和对象操作和 HTML 的表格元素来实现。示例代码如下:
```html
<table>
<thead>
<tr>
<th>姓名</th>
<th>工资</th>
<th>工时</th>
<th>时薪</th>
</tr>
</thead>
<tbody id="employeeTable"></tbody>
<tfoot>
<tr>
<td colspan="4" id="summary"></td>
</tr>
</tfoot>
</table>
```
```javascript
// 根据时薪降序排序
var sorted = salary.map(function(item) {
var h = hours.find(function(h) { return h.name === item.name; });
var hourlyPay = item.salary / h.hours;
return { name: item.name, salary: item.salary, hours: h.hours, hourlyPay: hourlyPay };
}).sort(function(a, b) {
return b.hourlyPay - a.hourlyPay;
});
// 取前2位员工
var top2 = sorted.slice(0, 2);
// 构造表格
var tbody = document.getElementById('employeeTable');
top2.forEach(function(item) {
var tr = document.createElement('tr');
var td1 = document.createElement('td');
td1.innerText = item.name;
var td2 = document.createElement('td');
td2.innerText = item.salary;
var td3 = document.createElement('td');
td3.innerText = item.hours;
var td4 = document.createElement('td');
td4.innerText = item.hourlyPay.toFixed(2);
tr.appendChild(td1);
tr.appendChild(td2);
tr.appendChild(td3);
tr.appendChild(td4);
tbody.appendChild(tr);
});
// 计算总薪资、总工时和平均时薪
var totalSalary = sorted.reduce(function(sum, item) { return sum + item.salary; }, 0);
var totalHours = sorted.reduce(function(sum, item) { return sum + item.hours; }, 0);
var averagePay = totalSalary / totalHours;
// 显示汇总信息
document.getElementById('summary').innerText = '总薪资:' + totalSalary + ',总工时:' + totalHours + ',平均时薪:' + averagePay.toFixed(2);
```
以上就是实现要求的代码示例,你可以根据需要进行修改和完善。
相关推荐
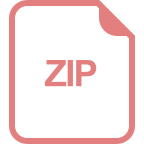
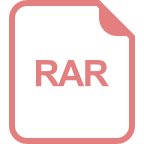














