java建造者模式代码
时间: 2023-04-02 22:02:13 浏览: 143
建造者模式是一种创建型设计模式,它允许您创建不同类型的对象,同时避免构造函数污染。以下是 Java 中的建造者模式示例代码:
public class Product {
private String property1;
private String property2;
private String property3;
public Product(ProductBuilder builder) {
this.property1 = builder.property1;
this.property2 = builder.property2;
this.property3 = builder.property3;
}
public String getProperty1() {
return property1;
}
public String getProperty2() {
return property2;
}
public String getProperty3() {
return property3;
}
public static class ProductBuilder {
private String property1;
private String property2;
private String property3;
public ProductBuilder() {}
public ProductBuilder withProperty1(String property1) {
this.property1 = property1;
return this;
}
public ProductBuilder withProperty2(String property2) {
this.property2 = property2;
return this;
}
public ProductBuilder withProperty3(String property3) {
this.property3 = property3;
return this;
}
public Product build() {
return new Product(this);
}
}
}
使用建造者模式创建对象的示例代码:
Product product = new Product.ProductBuilder()
.withProperty1("value1")
.withProperty2("value2")
.withProperty3("value3")
.build();
阅读全文
相关推荐
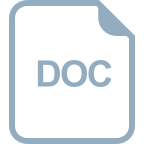
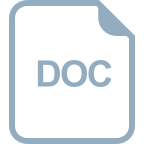
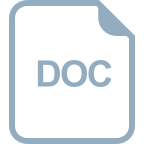
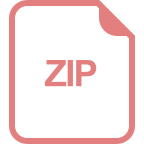

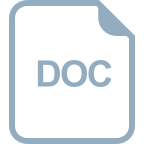
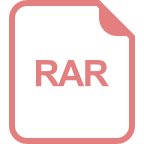
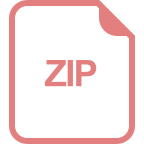
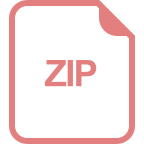
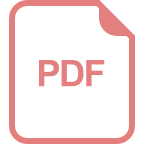
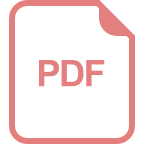
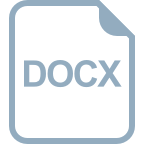
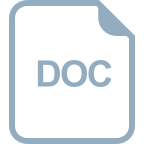





