static void readRes() //读取文件中的规则 { String filePath = "Res/res.txt"; //文件res.txt放在文件夹Res中 String line; //存放读取的每一行 try{ BufferedReader in = new BufferedReader(new FileReader(filePath)); line = in.readLine(); while(line != null) { List<String> arrayRule = new ArrayList<String>(); //每读取一行,创建一个List集合对象 listRule.add(arrayRule); //将创建的List集合对象添加到List集合listRule中 String[] txt = line.split(" "); //用空格分割读取的每条规则,并把其放入txt数组中 for(int i = 0;i < txt.length;i++) //for循环将每条规则中的每一项添加到List集合arrayRule中 { arrayRule.add(txt[i]); } line = in.readLine(); //读取下一行,即下一条规则 } in.close(); //关闭缓冲流 } catch(IOException iox){ System.out.println("读取文件" + filePath + "时出错!"); //读取文件异常 } }
时间: 2024-02-15 12:28:46 浏览: 17
这段代码是用来从文件中读取规则的。具体实现步骤为:
1. 定义一个字符串变量filePath,用于存放规则文件的路径。
2. 定义一个变量line,用于存放读取的每一行规则。
3. 使用BufferedReader类读取文件内容,其中FileReader类用于读取文件,BufferedReader类用于提高读取效率。
4. 定义一个List集合对象arrayRule,用于存储每条规则中的每一项。
5. 将arrayRule添加到另一个List集合对象listRule中,即将每条规则添加到一个集合中。
6. 使用split方法将每条规则用空格分割成多个字符串,将其放入txt数组中。
7. 使用for循环将每个txt字符串添加到arrayRule集合中。
8. 读取下一行规则,直到读取完整个文件。
9. 关闭缓冲流。
总的来说,这段代码实现了从文件中读取规则的功能,并将每条规则存储到了一个List集合中,方便后续的规则匹配和推理。
相关问题
readFile:fail permission denied, open ../../static/models/无标题.gltf
如果在uniapp微信小程序中加载本地gltf模型文件时遇到"readFile:fail permission denied, open"错误,这是因为微信小程序的安全机制限制了对本地文件的访问权限。
为了解决这个问题,你可以将模型文件放置在uniapp项目的静态资源目录中,比如`static/models`。
然后,使用相对路径加载模型文件。示例代码如下:
```javascript
const filePath = 'static/models/无标题.gltf';
wx.getFileSystemManager().readFile({
filePath: filePath,
encoding: 'utf8',
success: function (res) {
const gltfContent = res.data;
// 在这里处理 gltf 文件的内容
},
fail: function (res) {
console.log(res);
}
});
```
请确保替换示例代码中的`filePath`为你实际的模型文件路径。
通过使用`wx.getFileSystemManager().readFile`方法,并指定正确的相对路径,你可以读取并处理本地的gltf模型文件。
希望能帮到你!如果还有其他问题,请随时提问。
string.gsub(filepath, "^/", "")
string.gsub(filepath, "^/", "")是一个Lua字符串操作函数,用于将字符串中的指定模式替换为指定的内容。在这个例子中,它的作用是将filepath字符串中开头的斜杠("/")替换为空字符串("")。
具体来说,^表示匹配字符串的开头,/表示斜杠字符。所以,"^/"表示匹配以斜杠开头的字符串。通过将其替换为空字符串,就可以去掉filepath字符串开头的斜杠。
举个例子,如果filepath为"/path/to/file.txt",那么使用string.gsub(filepath, "^/", "")后,结果将变为"path/to/file.txt"。
相关推荐
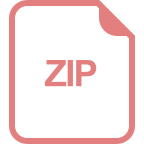
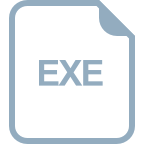
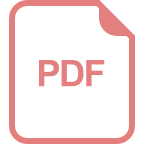













