使用c++设计一个菜鸟驿站系统
时间: 2023-12-10 20:38:50 浏览: 195
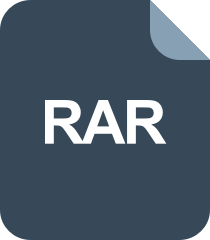
c++课程设计 学生选课系统
好的,以下是一个简单的菜鸟驿站系统的设计:
1. 定义数据结构
首先,我们需要定义数据结构来存储包裹和快递员信息。我们可以使用结构体来表示它们:
```c++
// 包裹信息结构体
struct Package {
int id; // 包裹编号
string sender; // 寄件人姓名
string receiver; // 收件人姓名
string address; // 收件地址
bool isDelivered; // 是否已送达
};
// 快递员信息结构体
struct Courier {
int id; // 快递员编号
string name; // 快递员姓名
bool isAvailable; // 快递员是否可用
};
```
2. 实现菜鸟驿站类
接下来,我们可以实现一个 `CourierStation` 类来管理包裹和快递员。该类可以包含以下成员变量和方法:
```c++
class CourierStation {
private:
vector<Package> packages; // 包裹列表
vector<Courier> couriers; // 快递员列表
public:
// 添加包裹
void addPackage(const Package& package);
// 删除包裹
void removePackage(int packageId);
// 更新包裹状态
void updatePackageStatus(int packageId, bool isDelivered);
// 添加快递员
void addCourier(const Courier& courier);
// 删除快递员
void removeCourier(int courierId);
// 查找空闲快递员
int findAvailableCourier();
// 显示所有包裹和快递员信息
void display();
};
```
3. 实现菜鸟驿站类的方法
下面我们来实现 `CourierStation` 类的方法:
```c++
void CourierStation::addPackage(const Package& package) {
packages.push_back(package);
}
void CourierStation::removePackage(int packageId) {
for (auto iter = packages.begin(); iter != packages.end(); iter++) {
if (iter->id == packageId) {
packages.erase(iter);
break;
}
}
}
void CourierStation::updatePackageStatus(int packageId, bool isDelivered) {
for (auto& package : packages) {
if (package.id == packageId) {
package.isDelivered = isDelivered;
break;
}
}
}
void CourierStation::addCourier(const Courier& courier) {
couriers.push_back(courier);
}
void CourierStation::removeCourier(int courierId) {
for (auto iter = couriers.begin(); iter != couriers.end(); iter++) {
if (iter->id == courierId) {
couriers.erase(iter);
break;
}
}
}
int CourierStation::findAvailableCourier() {
for (auto& courier : couriers) {
if (courier.isAvailable) {
courier.isAvailable = false;
return courier.id;
}
}
return -1; // 没有空闲快递员
}
void CourierStation::display() {
cout << "Packages:" << endl;
for (auto& package : packages) {
cout << "ID: " << package.id << ", Sender: " << package.sender << ", Receiver: " << package.receiver << ", Address: " << package.address << ", Status: " << (package.isDelivered ? "Delivered" : "Undelivered") << endl;
}
cout << endl;
cout << "Couriers:" << endl;
for (auto& courier : couriers) {
cout << "ID: " << courier.id << ", Name: " << courier.name << ", Status: " << (courier.isAvailable ? "Available" : "Unavailable") << endl;
}
cout << endl;
}
```
4. 测试
最后,我们可以编写一个简单的测试程序来测试 `CourierStation` 类的功能:
```c++
int main() {
CourierStation station;
// 添加包裹
Package package1 = {1, "Alice", "Bob", "123 Main St", false};
Package package2 = {2, "Charlie", "David", "456 Main St", false};
station.addPackage(package1);
station.addPackage(package2);
// 添加快递员
Courier courier1 = {1, "John", true};
Courier courier2 = {2, "Mary", true};
station.addCourier(courier1);
station.addCourier(courier2);
// 查找空闲快递员
int courierId = station.findAvailableCourier();
if (courierId != -1) {
cout << "Assign package 1 to courier " << courierId << endl;
station.updatePackageStatus(1, true);
station.display();
}
// 删除快递员和包裹
station.removeCourier(1);
station.removePackage(2);
station.display();
return 0;
}
```
输出结果应为:
```
Assign package 1 to courier 1
Packages:
ID: 1, Sender: Alice, Receiver: Bob, Address: 123 Main St, Status: Delivered
ID: 2, Sender: Charlie, Receiver: David, Address: 456 Main St, Status: Undelivered
Couriers:
ID: 1, Name: John, Status: Unavailable
ID: 2, Name: Mary, Status: Available
Packages:
ID: 1, Sender: Alice, Receiver: Bob, Address: 123 Main St, Status: Delivered
Couriers:
ID: 2, Name: Mary, Status: Available
```
阅读全文
相关推荐
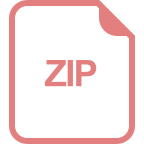
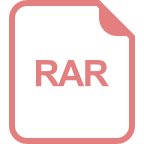












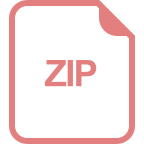
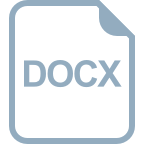
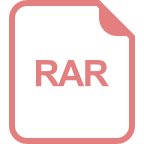
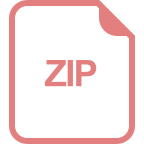