C++ 判断数据是否以 \n 结尾
时间: 2023-12-20 17:03:44 浏览: 33
可以使用 C++ 中的 `std::string` 类型的 `std::string::back()` 函数来判断一个字符串是否以 `\n` 结尾,示例代码如下:
```c++
#include <iostream>
#include <string>
int main() {
std::string str = "Hello world\n";
if (str.back() == '\n') {
std::cout << "The string ends with \\n" << std::endl;
} else {
std::cout << "The string does not end with \\n" << std::endl;
}
return 0;
}
```
输出结果为:
```
The string ends with \n
```
相关问题
QT socket如何判断数据接收完毕
在QT中使用Socket接收数据时,可以考虑以下两种方式来判断数据接收完毕:
1. 固定数据长度接收
如果发送方在发送数据时,已经确定了数据的长度,那么接收方可以根据这个长度来判断数据是否接收完毕。例如,发送方发送了一个100字节的字符串,接收方可以使用如下代码来接收数据,当接收到100字节的数据时,就可以判断数据接收完毕:
```c++
// 接收数据
char buffer[100];
int len = 0;
while (len < 100) {
int bytes = socket->read(buffer + len, 100 - len);
if (bytes == -1) {
// 发生错误
break;
} else if (bytes == 0) {
// 连接已关闭
break;
} else {
len += bytes;
}
}
if (len == 100) {
// 数据接收完毕
}
```
2. 按照特定标志接收
如果发送方在发送数据时,没有确定数据的长度,那么接收方可以使用特定的标志来判断数据是否接收完毕。例如,发送方在每条数据的结尾添加了一个换行符"\n",接收方可以使用如下代码来接收数据,当接收到换行符时,就可以判断数据接收完毕:
```c++
// 接收数据
QString buffer;
while (true) {
QByteArray data = socket->readLine();
if (data.isEmpty()) {
// 连接已关闭
break;
} else {
buffer.append(data);
if (buffer.endsWith("\n")) {
// 数据接收完毕
break;
}
}
}
```
以上是两种常用的判断数据接收完毕的方式,根据实际应用场景,可以选择合适的方式来判断数据接收完毕。
c++读文件夹里面的raw数据
C语言中可以使用标准库中的<dirent.h>来读取文件夹中的文件。下面是一个示例代码:
```c
#include <stdio.h>
#include <dirent.h>
#include <stdlib.h>
#include <string.h>
void readFolder(const char* folderPath) {
DIR* folder;
struct dirent* entry;
// 打开文件夹
folder = opendir(folderPath);
if (folder == NULL) {
printf("无法打开文件夹\n");
exit(1);
}
// 读取文件夹中的文件
while ((entry = readdir(folder)) != NULL) {
// 若是文件
if (entry->d_type == DT_REG) {
// 判断文件名是否以raw结尾
const char* ext = ".raw";
int len = strlen(entry->d_name);
if (len > 4 && strcmp(entry->d_name + len - 4, ext) == 0) {
printf("找到.raw文件:%s\n", entry->d_name);
// 进行相应处理或操作
}
}
}
// 关闭文件夹
closedir(folder);
}
int main() {
const char* folderPath = "路径/文件夹名";
readFolder(folderPath);
return 0;
}
```
以上代码使用了`opendir()`函数打开文件夹,然后使用`readdir()`函数读取文件夹中的文件。其中的`entry->d_type`用于判断是否为文件,`entry->d_name`用于获取文件名。通过判断文件名的后缀是否为".raw"来筛选出需要的文件。
你需要将代码中的`路径/文件夹名`替换为实际的文件夹路径。运行程序后,将会逐个输出找到的".raw"文件名。你可以在相应处理或操作的部分添加你需要的功能。
希望对你有帮助!
相关推荐
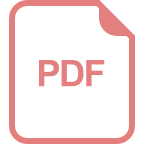












