c指针删除字符串空格
时间: 2023-07-26 11:04:45 浏览: 60
可以通过以下步骤来删除字符串中的空格:
1. 定义一个指针变量p,指向字符串的首地址。
2. 定义一个变量count,记录非空格字符的个数。
3. 循环遍历字符串中的每一个字符,直到遇到字符串结束符'\0'。
4. 判断当前字符是否为空格字符,如果不是,则将该字符复制到p所指向的地址,并将p向后移动一位,同时将count加1。
5. 如果是空格字符,则直接跳过,不复制。
6. 循环结束后,在新字符串的末尾添加字符串结束符'\0'。
7. 最后返回新字符串的首地址。
下面是一段示例代码:
```
#include <stdio.h>
#include <string.h>
#include <ctype.h>
char* remove_spaces(char* str) {
char* p = str;
int count = 0;
while (*str) {
if (!isspace(*str)) {
*p++ = *str;
count++;
}
str++;
}
*p = '\0';
return str - count;
}
int main() {
char str[] = " hello world ";
printf("Original string: %s\n", str);
char* new_str = remove_spaces(str);
printf("New string: %s\n", new_str);
return 0;
}
```
输出结果为:
```
Original string: hello world
New string: helloworld
```
相关推荐
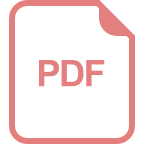
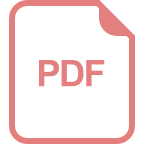
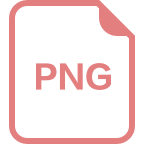
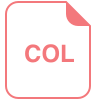
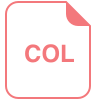
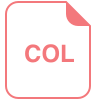
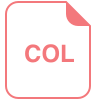
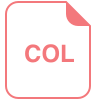









