c语言统计字符串空格多少个
时间: 2023-12-12 20:02:30 浏览: 118
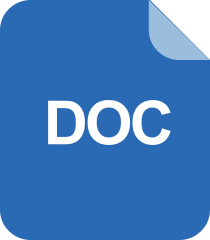
统计字符串的字母空格个数
很高兴回答你的问题!对于统计字符串中空格的数量,在C语言中我们可以使用循环逐个字符比对的方法,以下是一个简单的代码示例:
```c
#include <stdio.h>
#include <string.h>
int count_spaces(char* str) {
int count = 0;
for (int i = 0; i < strlen(str); i++) {
if (str[i] == ' ') {
count++;
}
}
return count;
}
int main() {
char str[] = "Hello world, how are you?";
int spaces = count_spaces(str);
printf("Number of spaces in string: %d", spaces);
return 0;
}
```
以上代码中,我们首先定义了一个函数 `count_spaces` ,它的输入是一个字符指针 `str` ,输出是整型变量 `count` ,代表字符串中空格的数量。在函数中,我们使用了一个循环逐个比对字符串中的字符,如果某个字符是空格,我们将计数器 `count` 的值加一。最后,在 `main` 函数中,我们定义了一个测试用的字符串 `str` ,并调用了 `count_spaces` 函数,将空格的数量存放在变量 `spaces` 中并输出。
阅读全文
相关推荐
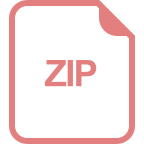
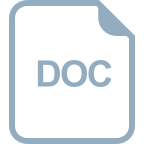













