c语言统计字符串长度中间有空格怎么办
时间: 2024-10-01 15:13:00 浏览: 33
在 C 语言中,如果你需要统计包含空格的字符串长度,可以使用标准库函数 `strlen()`。但是,`strlen()` 只计算连续字符的数量,包括空格。如果你想排除空格不算入长度,你需要遍历字符串并手动计数。
下面是一个简单的示例:
```c
#include <stdio.h>
#include <string.h>
size_t count_chars(const char *str) {
size_t length = 0;
while (*str) { // 遍历直到遇到空字符 '\0'
if (*str != ' ') { // 如果不是空格,则增加长度
length++;
}
str++; // 移动指针到下一个字符
}
return length;
}
int main() {
const char *input = "Hello, World!";
size_t len = count_chars(input);
printf("实际有效字符长度: %zu\n", len);
return 0;
}
```
在这个例子中,`count_chars()` 函数会返回输入字符串中非空格字符的实际长度。
相关问题
c语言统计字符串出现的次数
可以使用循环遍历字符串,每次判断当前字符是否为目标字符,如果是则计数器加一。具体代码如下:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[100];
char target;
int count = 0;
printf("请输入字符串:");
scanf("%s", str);
printf("请输入要统计的字符:");
scanf(" %c", &target);
for (int i = 0; i < strlen(str); i++) {
if (str[i] == target) {
count++;
}
}
printf("%c 出现的次数为:%d\n", target, count);
return 0;
}
```
这里使用了 `strlen()` 函数获取字符串长度,需要包含头文件 `string.h`。注意在读取目标字符时需要加一个空格,避免读取到上一个输入缓存中的回车符。
c语言输入一个长度小于100的字符串(字符串中允许包含空格),统计该字符串中大写字母,小写字母,数字字符及其它字符的数量。
可以使用C语言中的字符数组和循环语句来实现该程序。具体步骤如下:
1. 定义一个字符数组来存储输入的字符串,长度为100(包括字符串末尾的'\0')。
2. 使用fgets()函数从标准输入(stdin)中读取输入的字符串,并将其存储到字符数组中。
3. 定义四个变量(分别表示大写字母、小写字母、数字字符和其它字符的数量),并初始化为0。
4. 使用for循环遍历字符数组中的每个字符,根据其ASCII码值判断它属于哪一类字符,并将相应的计数器加1。
5. 输出四个计数器的值。
下面是示例代码:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
int upper = 0, lower = 0, digit = 0, other = 0;
printf("请输入一个字符串(长度小于100):\n");
fgets(str, 100, stdin);
for (int i = 0; str[i] != '\0'; i++) {
if (isupper(str[i])) {
upper++;
} else if (islower(str[i])) {
lower++;
} else if (isdigit(str[i])) {
digit++;
} else {
other++;
}
}
printf("大写字母:%d\n", upper);
printf("小写字母:%d\n", lower);
printf("数字字符:%d\n", digit);
printf("其它字符:%d\n", other);
return 0;
}
```
注意:在统计字符数量时,可以使用C标准库中的ctype.h头文件中的函数来判断字符类型。isupper()函数用于判断字符是否为大写字母,islower()函数用于判断字符是否为小写字母,isdigit()函数用于判断字符是否为数字字符。
阅读全文
相关推荐
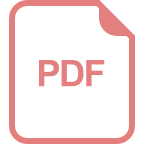
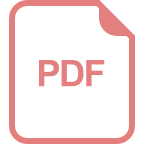
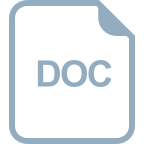
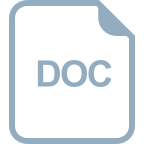












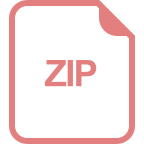