c语言写一下字符串长度冒泡
时间: 2024-10-21 22:17:44 浏览: 28
在 C 语言中,你可以使用标准库函数 `strlen()` 来直接计算字符串的长度,因为它已经实现了这个功能。`strlen()` 函数接受一个 null-终止的字符数组作为输入,并返回从第一个字符到null字符之间的字节数,即字符串长度。但是如果你想通过冒泡排序的方式“模拟”字符串长度的计算,那实际上并不合适,因为冒泡排序主要用于对整数列表进行排序,而不是用于字符串处理。
不过,如果你真的想要演示冒泡排序算法并且顺便统计字符个数(忽略空格等非字母数字字符),可以编写一个简单的函数,如下所示:
```c
#include <stdio.h>
#include <ctype.h>
int bubble_sort_length(char str[]) {
int len = 0;
int n = strlen(str);
for (int i = 0; i < n - 1; ++i) {
for (int j = 0; j < n - i - 1; ++j) {
if (!isspace(str[j]) && !ispunct(str[j])) { // 忽略空格和标点
++len;
}
if (str[j] == '\0') break; // 如果遇到null字符,说明已遍历完有效字符
}
}
return len;
}
int main() {
char test_str[] = "Hello, World! This is a test.";
int length = bubble_sort_length(test_str);
printf("The effective string length (ignoring spaces and punctuation) is %d.\n", length);
return 0;
}
```
在这个示例里,我们用冒泡排序的思想来遍历字符串,但实际上并没有真正进行排序操作。`bubble_sort_length` 函数通过比较字符并跳过空格和标点来计数有效字符的数量。
阅读全文
相关推荐
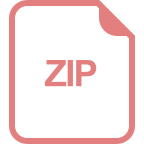
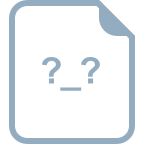
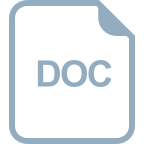
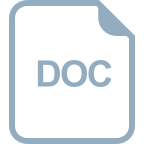
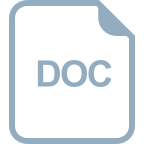
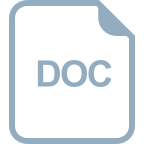
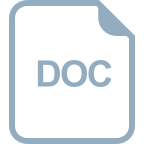
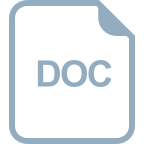
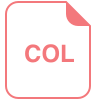








