哈夫曼编码的java代码
时间: 2023-07-19 19:17:39 浏览: 111
以下是一个简单的 Java 实现哈夫曼编码的代码示例:
```java
import java.util.PriorityQueue;
import java.util.Scanner;
public class HuffmanEncoder {
static class HuffmanNode implements Comparable<HuffmanNode> {
int frequency;
char c;
HuffmanNode left, right;
public int compareTo(HuffmanNode hn) {
return frequency - hn.frequency;
}
}
public static void printCode(HuffmanNode root, String s) {
if (root.left == null && root.right == null && Character.isLetter(root.c)) {
System.out.println(root.c + ":" + s);
return;
}
printCode(root.left, s + "0");
printCode(root.right, s + "1");
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("请输入字符串: ");
String str = sc.nextLine();
int[] freq = new int[26];
for (int i = 0; i < str.length(); i++) {
freq[str.charAt(i) - 'a']++;
}
PriorityQueue<HuffmanNode> q = new PriorityQueue<>();
for (char c = 'a'; c <= 'z'; c++) {
if (freq[c - 'a'] > 0) {
HuffmanNode hn = new HuffmanNode();
hn.c = c;
hn.frequency = freq[c - 'a'];
hn.left = null;
hn.right = null;
q.add(hn);
}
}
HuffmanNode root = null;
while (q.size() > 1) {
HuffmanNode x = q.peek();
q.poll();
HuffmanNode y = q.peek();
q.poll();
HuffmanNode f = new HuffmanNode();
f.frequency = x.frequency + y.frequency;
f.c = '-';
f.left = x;
f.right = y;
root = f;
q.add(f);
}
System.out.println("字符编码:");
printCode(root, "");
}
}
```
该代码首先读入一个字符串,然后计算每个字符出现的频率。接下来,使用优先队列实现了哈夫曼树的构建。最后,使用递归方法打印出每个字符的哈夫曼编码。
阅读全文
相关推荐
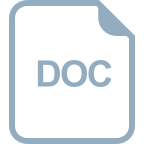
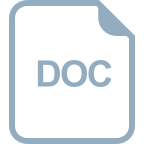
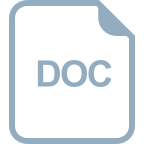




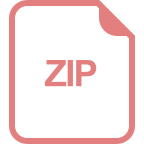



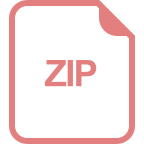

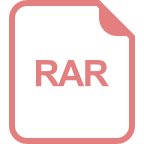

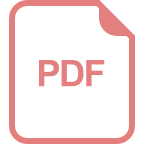
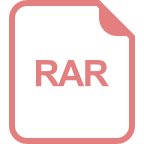
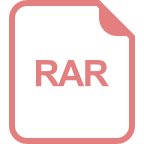