用javascript语言编写一个烟花程序
时间: 2023-08-31 16:34:00 浏览: 111
### 回答1:
以下是一个用JavaScript编写的基本烟花程序,它可以在HTML5 Canvas上绘制随机颜色的烟花,用鼠标点击屏幕触发烟花效果:
```html
<!DOCTYPE html>
<html>
<head>
<title>Fireworks Demo</title>
<style>
canvas {
background-color: black;
}
</style>
</head>
<body>
<canvas id="canvas"></canvas>
<script>
// 获取canvas元素和2D绘图上下文
const canvas = document.getElementById('canvas');
const ctx = canvas.getContext('2d');
// 设置canvas大小
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
// 烟花粒子类
class Particle {
constructor(x, y, color) {
this.x = x;
this.y = y;
this.color = color;
this.radius = 5;
this.vx = Math.random() * 6 - 3;
this.vy = Math.random() * 6 - 3;
this.alpha = 1;
}
// 绘制粒子
draw() {
ctx.globalAlpha = this.alpha;
ctx.beginPath();
ctx.arc(this.x, this.y, this.radius, 0, 2 * Math.PI);
ctx.fillStyle = this.color;
ctx.fill();
}
// 更新粒子状态
update() {
this.x += this.vx;
this.y += this.vy;
this.alpha -= 0.02;
this.radius -= 0.02;
}
// 是否消失
get isDead() {
return this.alpha <= 0 || this.radius <= 0;
}
}
// 烟花类
class Firework {
constructor(x, y) {
this.x = x;
this.y = y;
this.color = `rgb(${Math.random() * 255}, ${Math.random() * 255}, ${Math.random() * 255})`;
this.particles = [];
for (let i = 0; i < 50; i++) {
this.particles.push(new Particle(this.x, this.y, this.color));
}
}
// 绘制烟花
draw() {
this.particles.forEach(particle => particle.draw());
}
// 更新烟花状态
update() {
this.particles.forEach(particle => particle.update());
this.particles = this.particles.filter(particle => !particle.isDead);
}
// 是否消失
get isDead() {
return this.particles.length === 0;
}
}
// 存储烟花
const fireworks = [];
// 鼠标点击事件
canvas.addEventListener('click', function(e) {
fireworks.push(new Firework(e.clientX, e.clientY));
});
// 渲染循环
function loop() {
// 清除canvas
ctx.clearRect(0, 0, canvas.width, canvas.height);
// 更新和绘制烟花
fireworks.forEach(firework => {
firework.update();
firework.draw();
});
// 移除消失的烟花
fireworks = fireworks.filter(firework => !firework.isDead);
// 下一帧
requestAnimationFrame(loop);
}
### 回答2:
JavaScript是一种用于网页开发的脚本语言,可以用它编写一个简单的烟花程序。下面是一个基本的示例:
1. 创建HTML页面结构:
```html
<!DOCTYPE html>
<html>
<head>
<title>烟花程序</title>
<style>
#fireworks {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
overflow: hidden;
}
</style>
</head>
<body>
<div id="fireworks"></div>
<script src="fireworks.js"></script>
</body>
</html>
```
2. 创建JavaScript文件fireworks.js:
```javascript
// 获取页面上的烟花容器
var fireworksContainer = document.getElementById('fireworks');
// 初始化烟花
function Firework() {
// 烟花位置
this.x = Math.random() * fireworksContainer.offsetWidth;
this.y = fireworksContainer.offsetHeight;
// 烟花颜色
this.color = '#' + Math.floor(Math.random() * 16777215).toString(16);
// 烟花速度
this.speed = Math.random() * 5 + 1;
// 烟花升空
this.launch = function() {
var firework = document.createElement('div');
firework.style.position = 'absolute';
firework.style.width = '5px';
firework.style.height = '5px';
firework.style.borderRadius = '50%';
firework.style.backgroundColor = this.color;
firework.style.left = this.x + 'px';
firework.style.top = this.y + 'px';
fireworksContainer.appendChild(firework);
var that = this;
setInterval(function() {
that.y -= that.speed;
firework.style.top = that.y + 'px';
if (that.y <= 0) {
// 移除烟花
fireworksContainer.removeChild(firework);
clearInterval(this);
}
}, 20);
};
}
// 创建烟花
function createFirework() {
var firework = new Firework();
firework.launch();
setTimeout(createFirework, Math.random() * 2000 + 500);
}
// 延迟一段时间后开始创建烟花
setTimeout(createFirework, 1000);
```
以上是一个简单的烟花程序的实现。它会在页面上创建一个烟花容器,然后通过不断创建和移除烟花元素的方式模拟烟花升空和爆炸的效果。每个烟花的颜色、位置和速度都是随机生成的。程序会在页面加载完毕后延迟一段时间开始创建烟花,并且每次创建烟花的间隔时间也是随机的,以增加效果的多样性。
阅读全文
相关推荐
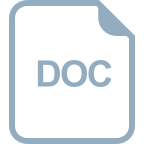
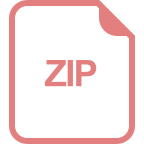
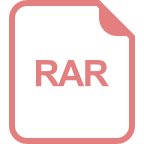
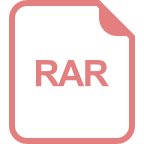
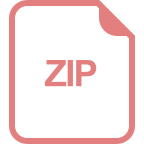
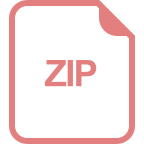
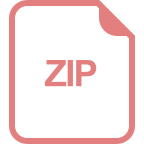
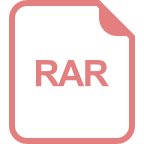
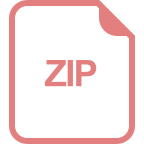
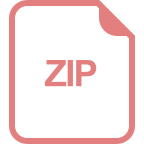
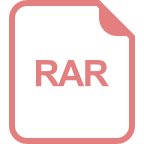
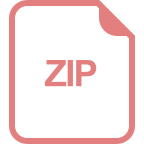
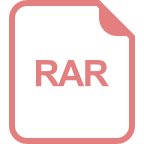
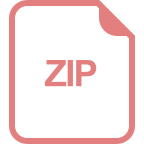
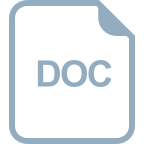
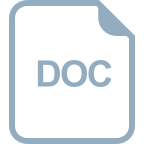