JDBC实现index.jsp页面用于显示用户名。显示“用户信息”超链接,点击该超链接跳转到userList.jsp。如果用户没有登录,在index.jsp页面中就显示一个用户登录的超链接。思路
时间: 2024-03-14 19:45:20 浏览: 93
首先,需要在index.jsp页面中判断用户是否已经登录。如果已经登录,则显示用户名和一个“用户信息”超链接,否则显示一个用户登录的超链接。
可以通过在session中存储登录状态来判断用户是否已经登录。如果用户已经登录,则可以从session中获取用户名并在页面中显示。如果用户没有登录,则可以在页面中显示一个登录超链接。
在index.jsp页面中,可以使用JSTL标签库来判断用户是否已经登录,例如:
```
<c:if test="${sessionScope.username != null}">
<p>Welcome, ${sessionScope.username}!</p>
<a href="userList.jsp">User Info</a>
</c:if>
<c:if test="${sessionScope.username == null}">
<a href="login.jsp">Login</a>
</c:if>
```
其中,`${sessionScope.username}`表示从session中获取用户名。
在点击“用户信息”超链接时,可以跳转到userList.jsp页面。在userList.jsp页面中,可以使用JDBC来查询并显示用户信息。例如:
```
<%
Connection conn = null;
PreparedStatement stmt = null;
ResultSet rs = null;
try {
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "root", "password");
stmt = conn.prepareStatement("SELECT * FROM users");
rs = stmt.executeQuery();
while (rs.next()) {
out.println("<p>" + rs.getString("username") + "</p>");
}
} catch (SQLException ex) {
ex.printStackTrace();
} finally {
if (rs != null) rs.close();
if (stmt != null) stmt.close();
if (conn != null) conn.close();
}
%>
```
其中,`jdbc:mysql://localhost:3306/mydatabase`表示数据库连接字符串,`root`表示数据库用户名,`password`表示数据库密码。你需要根据实际情况修改这些参数。查询的结果通过`out.println`方法输出到页面上。
阅读全文
相关推荐
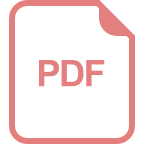
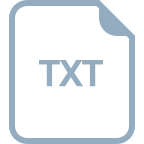
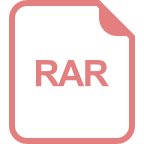
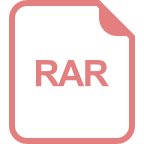
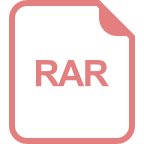
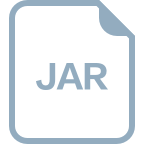
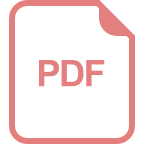
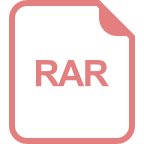
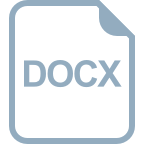
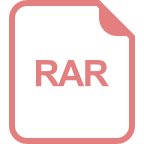
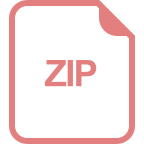
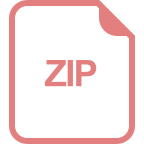
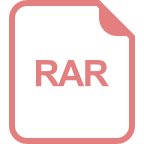