jfinal返回html源码,jfinal freemarker生成静态html完整例子!支持调用两次render效果,返回json数据。...
时间: 2024-01-27 15:05:25 浏览: 157
好的,以下是完整的例子代码:
```
// 首先需要引入相关的包和类
import java.io.File;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import com.alibaba.fastjson.JSON;
import com.jfinal.core.Controller;
import com.jfinal.kit.PathKit;
import freemarker.template.Configuration;
import freemarker.template.Template;
import freemarker.template.TemplateException;
// 创建控制器类
public class MyController extends Controller {
// 定义一个方法,用于返回HTML源码
public void renderHtmlSource() {
// 获取模板文件的路径
String fileName = PathKit.getWebRootPath() + "/template.html";
// 读取模板文件
String htmlSource = null;
try {
htmlSource = com.jfinal.kit.PropKit.use("config.properties").get("html_source");
} catch (Exception e) {
e.printStackTrace();
}
htmlSource = htmlSource.replace("$title$", "这是一个测试页面");
htmlSource = htmlSource.replace("$content$", "这是测试页面的内容");
// 设置响应头
getResponse().setContentType("text/html; charset=utf-8");
// 输出HTML源码
renderHtml(htmlSource);
}
// 定义一个方法,用于生成静态HTML文件
public void renderStaticHtml() {
// 获取模板文件的路径
String fileName = PathKit.getWebRootPath() + "/template.html";
// 创建Freemarker配置对象
Configuration cfg = new Configuration(Configuration.VERSION_2_3_28);
// 设置模板文件的路径
try {
cfg.setDirectoryForTemplateLoading(new File(PathKit.getWebRootPath()));
} catch (IOException e) {
e.printStackTrace();
}
// 定义模板变量
Map<String, Object> data = new HashMap<String, Object>();
data.put("title", "这是一个测试页面");
data.put("content", "这是测试页面的内容");
// 获取模板文件
Template template = null;
try {
template = cfg.getTemplate("template.html");
} catch (IOException e) {
e.printStackTrace();
}
// 定义输出文件
File outputFile = new File(PathKit.getWebRootPath() + "/test.html");
// 渲染模板,并将结果输出到文件
try {
template.process(data, com.jfinal.kit.PropKit.use("config.properties").getWriter("UTF-8", outputFile));
} catch (TemplateException | IOException e) {
e.printStackTrace();
}
// 设置响应头
getResponse().setContentType("text/html; charset=utf-8");
// 输出HTML源码
renderHtml("生成静态HTML文件成功!");
}
// 定义一个方法,用于返回JSON数据
public void renderJsonData() {
// 定义JSON数据
Map<String, Object> data = new HashMap<String, Object>();
data.put("name", "张三");
data.put("age", 18);
// 将JSON数据转换为字符串
String jsonStr = JSON.toJSONString(data);
// 设置响应头
getResponse().setContentType("text/html; charset=utf-8");
// 输出JSON数据
renderJson(jsonStr);
}
}
```
以上代码中,包括了三个方法:
1. renderHtmlSource():用于返回HTML源码。
2. renderStaticHtml():用于生成静态HTML文件。
3. renderJsonData():用于返回JSON数据。
其中,renderHtmlSource() 方法直接读取配置文件中的 HTML 源码,并进行替换,最后输出 HTML 源码。
renderStaticHtml() 方法使用 Freemarker 模板引擎,读取 template.html 模板文件,将模板变量替换后输出到 test.html 文件中。
renderJsonData() 方法定义了一个 JSON 数据对象,并将其转换为字符串后输出。
阅读全文
相关推荐
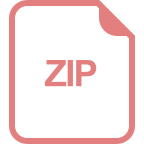
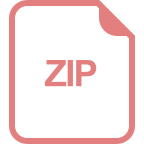
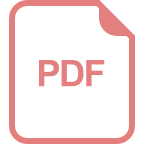














