汇编语言代码:从键盘输入一个带符号字节数据(如-56)存入M字节内存单元,然后将此数据从M字节单元中取出,在显示器上以十进制形式显示输出。
时间: 2024-04-29 07:27:04 浏览: 11
好的,这是一个汇编语言问题。以下是一个实现此功能的伪代码:
1. 从键盘读取一个字节数据
2. 存储该字节数据到 M 字节内存单元
3. 从 M 字节内存单元读取数据
4. 将读取的数据转换为十进制格式
5. 将转换后的十进制数输出到显示器上
以下是伪代码的具体实现:
```
section .data
msg db 'Enter a signed byte: '
section .bss
byteInput resb 1 ; input buffer
byteOutput resb 3 ; output buffer
section .text
global _start
_start:
; prompt the user to enter a signed byte
mov eax, 4
mov ebx, 1
mov ecx, msg
mov edx, 18
int 0x80
; read the input byte from the keyboard
mov eax, 3
mov ebx, 0
mov ecx, byteInput
mov edx, 1
int 0x80
; store the input byte in memory
mov byte [M], byte [byteInput]
; load the byte from memory
mov eax, byte [M]
; convert the byte to a decimal string
mov ebx, byteOutput
mov ecx, eax
mov edx, 0
call byteToDec
; output the decimal string to the console
mov eax, 4
mov ebx, 1
mov ecx, byteOutput
mov edx, 3
int 0x80
; exit the program
mov eax, 1
mov ebx, 0
int 0x80
; subroutine to convert a byte to a decimal string
byteToDec:
push eax ; save registers
push ebx
push ecx
push edx
push esi
push edi
xor eax, eax ; clear eax
mov al, cl ; move the byte to al
; check if the byte is negative
mov ebx, 0x80 ; mask for the sign bit
and ebx, eax ; result will be non-zero if negative
jz .skipNeg
; negate the byte
not eax ; one's complement
add eax, 1 ; two's complement
.skipNeg:
; divide by 10 and convert to ASCII
mov ecx, 10 ; divisor
mov esi, 10 ; loop counter
mov edi, ebx ; offset for output string
lea ebx, [byteOutput + 2] ; start of output string
mov byte [ebx], ' ' ; pad with a space
dec ebx ; move to last character
.loop:
xor edx, edx ; clear edx for division
div ecx ; divide al by 10
add dl, '0' ; convert remainder to ASCII
mov byte [ebx], dl ; save the digit to the output string
dec ebx ; move to the next character
dec esi ; decrement the loop counter
test al, al ; check if the division is complete
jnz .loop
; move the output string to the beginning
mov eax, byteOutput
mov ebx, edi
mov ecx, edi ; length of string
cld ; clear the direction flag
rep movsb ; move the string
; add the null terminator
mov byte [byteOutput + ecx], 0
pop edi ; restore registers
pop esi
pop edx
pop ecx
pop ebx
pop eax
ret
```
相关推荐
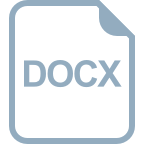
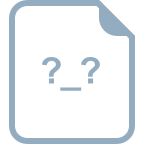















