假定算数表达式的值均为非负整数常数,不包含变量、小 数和字符常量。 用C++,试设计一个算术四则运算表达式求值的简单计算器。 基本要求: 1、 操作数均为非负整数常数,操作符仅为+、-、*、/、(、); 2、 编写 main 函数进行测试。给出详细注释
时间: 2023-11-08 13:03:56 浏览: 167
好的,我知道了。对于你的第一个问题,以下是简单计算器的C语言代码,已详细注释。
```
#include <stdio.h>
#include <stdlib.h> // 包含函数 atof 和 exit 的头文件
#define MAXOP 100 // 操作数或运算符的最大长度
#define NUMBER '0' // 标识找到一个数
int getop(char[]); // 获取下一个运算符或操作数
void push(double); // 压入操作数栈
double pop(void); // 弹出并返回栈顶操作数
int main()
{
int type;
double op2;
char s[MAXOP];
while ((type = getop(s)) != EOF) {
switch (type) {
case NUMBER:
push(atof(s)); // 将 s 转换为 double 类型并压入栈中
break;
case '+':
push(pop() + pop()); // 弹出栈顶两个操作数相加并压入栈中
break;
case '-':
op2 = pop();
push(pop() - op2); // 弹出栈顶两个操作数相减并压入栈中
break;
case '*':
push(pop() * pop()); // 弹出栈顶两个操作数相乘并压入栈中
break;
case '/':
op2 = pop();
if (op2 == 0.0) {
printf("error: zero divisor\n");
exit(1); // 除数为 0,退出程序
}
push(pop() / op2); // 弹出栈顶两个操作数相除并压入栈中
break;
case '\n':
printf("result: %.8g\n", pop()); // 输出计算结果
break;
default:
printf("error: unknown command %s\n", s);
exit(1); // 遇到未知命令,退出程序
break;
}
}
return 0;
}
#define MAXVAL 100 // 操作数栈的最大深度
int sp = 0; // 操作数栈的下一个空闲位置
double val[MAXVAL]; // 操作数栈
void push(double f)
{
if (sp < MAXVAL)
val[sp++] = f;
else
printf("error: stack full, can't push %g\n", f);
}
double pop(void)
{
if (sp > 0)
return val[--sp];
else {
printf("error: stack empty\n");
exit(1); // 操作数栈已空,退出程序
}
}
#include <ctype.h>
int getch(void);
void ungetch(int);
int getop(char s[])
{
int i, c;
while ((s[0] = c = getch()) == ' ' || c == '\t')
; // 跳过空格和制表符
s[1] = '\0';
if (!isdigit(c) && c != '.')
return c; // 不是数也不是小数点,就是运算符
i = 0;
if (isdigit(c)) // 匹配整数部分
while (isdigit(s[++i] = c = getch()))
;
if (c == '.') // 匹配小数部分
while (isdigit(s[++i] = c = getch()))
;
s[i] = '\0';
if (c != EOF)
ungetch(c);
return NUMBER; // 标识找到了数
}
#define BUFSIZE 100 // 用于 ungetch 函数的缓冲区大小
char buf[BUFSIZE]; // 缓冲区
int bufp = 0; // 标识下一个空闲位置
int getch(void) // 取一个字符(可能是压回的字符)
{
return (bufp > 0) ? buf[--bufp] : getchar();
}
void ungetch(int c) // 把字符压回到输入中
{
if (bufp >= BUFSIZE)
printf("ungetch: too many characters\n");
else
buf[bufp++] = c;
}
```
希望这份代码能帮到你。
阅读全文
相关推荐
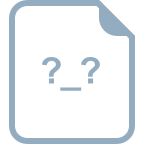

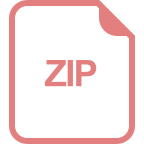
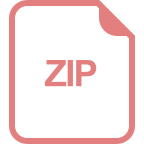
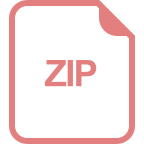
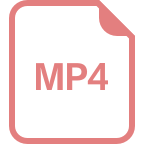
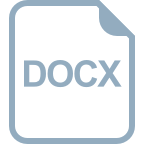
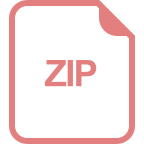
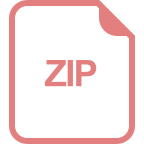
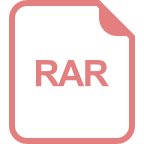
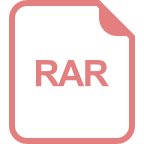
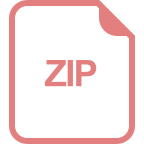