帮我写一段vc五彩气球的代码
时间: 2023-07-10 13:10:28 浏览: 168
好的,以下是一个简单的VC++五彩气球代码示例:
```cpp
#include <windows.h>
#include <cstdlib>
#include <ctime>
#include <cmath>
#include <vector>
using namespace std;
// 窗口类名
const char g_szClassName[] = "MyWindowClass";
// 气球结构体
struct Balloon {
int x, y; // 气球位置
int r; // 气球半径
int dx, dy; // 气球移动速度
COLORREF color; // 气球颜色
};
// 全局变量
vector<Balloon> g_balloons; // 气球数组
int g_bkWidth, g_bkHeight; // 背景大小
// 生成随机颜色
COLORREF RandomColor() {
return RGB(rand() % 256, rand() % 256, rand() % 256);
}
// 初始化气球
void InitBalloons() {
g_balloons.clear();
for (int i = 0; i < 20; i++) {
Balloon balloon;
balloon.x = rand() % (g_bkWidth - 100) + 50;
balloon.y = rand() % (g_bkHeight - 100) + 50;
balloon.r = rand() % 30 + 20;
balloon.dx = rand() % 5 + 1;
balloon.dy = rand() % 5 + 1;
balloon.color = RandomColor();
g_balloons.push_back(balloon);
}
}
// 绘制气球
void DrawBalloon(HDC hdc, Balloon &balloon) {
HBRUSH hBrush = CreateSolidBrush(balloon.color);
HBRUSH hOldBrush = (HBRUSH)SelectObject(hdc, hBrush);
Ellipse(hdc, balloon.x - balloon.r, balloon.y - balloon.r, balloon.x + balloon.r, balloon.y + balloon.r);
SelectObject(hdc, hOldBrush);
DeleteObject(hBrush);
}
// 窗口过程函数
LRESULT CALLBACK WndProc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam) {
switch (msg) {
case WM_CREATE:
// 获取窗口大小
RECT rc;
GetClientRect(hwnd, &rc);
g_bkWidth = rc.right - rc.left;
g_bkHeight = rc.bottom - rc.top;
// 初始化随机数种子
srand(time(NULL));
// 初始化气球
InitBalloons();
// 设置定时器
SetTimer(hwnd, 1, 10, NULL);
break;
case WM_PAINT:
// 绘制背景
PAINTSTRUCT ps;
HDC hdc = BeginPaint(hwnd, &ps);
HBRUSH hBrush = CreateSolidBrush(RGB(255, 255, 255));
FillRect(hdc, &ps.rcPaint, hBrush);
DeleteObject(hBrush);
// 绘制气球
for (int i = 0; i < g_balloons.size(); i++) {
DrawBalloon(hdc, g_balloons[i]);
}
EndPaint(hwnd, &ps);
break;
case WM_TIMER:
// 移动气球
for (int i = 0; i < g_balloons.size(); i++) {
Balloon &balloon = g_balloons[i];
balloon.x += balloon.dx;
balloon.y += balloon.dy;
// 碰撞检测
if (balloon.x - balloon.r < 0 || balloon.x + balloon.r > g_bkWidth) {
balloon.dx = -balloon.dx;
}
if (balloon.y - balloon.r < 0 || balloon.y + balloon.r > g_bkHeight) {
balloon.dy = -balloon.dy;
}
}
// 重绘窗口
InvalidateRect(hwnd, NULL, FALSE);
break;
case WM_DESTROY:
// 销毁定时器
KillTimer(hwnd, 1);
// 退出程序
PostQuitMessage(0);
break;
default:
return DefWindowProc(hwnd, msg, wParam, lParam);
}
return 0;
}
// WinMain函数
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow) {
// 创建窗口类
WNDCLASSEX wc;
wc.cbSize = sizeof(WNDCLASSEX);
wc.style = 0;
wc.lpfnWndProc = WndProc;
wc.cbClsExtra = 0;
wc.cbWndExtra = 0;
wc.hInstance = hInstance;
wc.hIcon = LoadIcon(NULL, IDI_APPLICATION);
wc.hCursor = LoadCursor(NULL, IDC_ARROW);
wc.hbrBackground = (HBRUSH)(COLOR_WINDOW + 1);
wc.lpszMenuName = NULL;
wc.lpszClassName = g_szClassName;
wc.hIconSm = LoadIcon(NULL, IDI_APPLICATION);
if (!RegisterClassEx(&wc)) {
MessageBox(NULL, "窗口类注册失败!", "错误", MB_ICONERROR);
return 0;
}
// 创建窗口
HWND hwnd = CreateWindowEx(WS_EX_CLIENTEDGE, g_szClassName, "五彩气球", WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT, CW_USEDEFAULT, 800, 600, NULL, NULL, hInstance, NULL);
if (hwnd == NULL) {
MessageBox(NULL, "窗口创建失败!", "错误", MB_ICONERROR);
return 0;
}
// 显示窗口
ShowWindow(hwnd, nCmdShow);
UpdateWindow(hwnd);
// 消息循环
MSG msg;
while (GetMessage(&msg, NULL, 0, 0)) {
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return (int)msg.wParam;
}
```
这个代码会在窗口中绘制20个随机颜色的气球,气球会在窗口中自由移动,碰到窗口边缘会反弹。如果需要添加音效和背景音乐,您可以使用WinAPI提供的多媒体函数来实现。
阅读全文
相关推荐
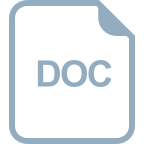
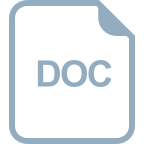
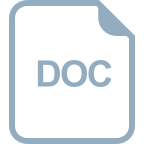
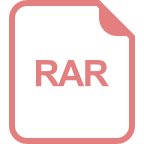
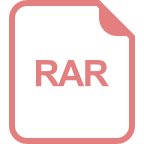
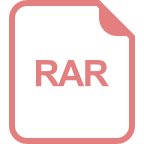
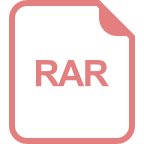
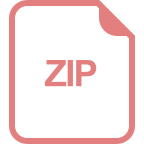
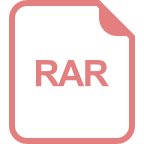
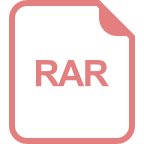
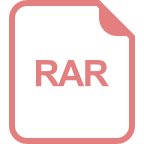