vue实现数据库一张表的增删改查
时间: 2023-08-30 20:12:33 浏览: 77
首先,您需要使用Vue的HTTP模块或者Axios等工具与后端接口进行交互,来实现对数据库的增删改查操作。以下是一个简单的示例:
假设您的后端接口为:
```
GET /api/users // 获取所有用户
GET /api/users/:id // 获取指定用户
POST /api/users // 新增用户
PUT /api/users/:id // 更新指定用户
DELETE /api/users/:id // 删除指定用户
```
您可以使用Vue的组件来实现增删改查功能:
```html
<template>
<div>
<div v-for="user in users" :key="user.id">
<span>{{ user.name }}</span>
<button @click="editUser(user)">编辑</button>
<button @click="deleteUser(user)">删除</button>
</div>
<button @click="addUser()">新增用户</button>
<div v-if="showEditDialog">
<input v-model="editingUser.name">
<button @click="saveUser()">保存</button>
<button @click="cancelEdit()">取消</button>
</div>
</div>
</template>
<script>
import axios from 'axios'
export default {
data() {
return {
users: [], // 所有用户
editingUser: null, // 当前编辑的用户
showEditDialog: false, // 是否显示编辑对话框
}
},
mounted() {
this.getUsers()
},
methods: {
async getUsers() {
const res = await axios.get('/api/users')
this.users = res.data
},
async getUser(id) {
const res = await axios.get(`/api/users/${id}`)
return res.data
},
async addUser() {
const res = await axios.post('/api/users', { name: '新用户' })
this.users.push(res.data)
},
async editUser(user) {
this.editingUser = await this.getUser(user.id)
this.showEditDialog = true
},
async saveUser() {
await axios.put(`/api/users/${this.editingUser.id}`, this.editingUser)
this.editingUser = null
this.showEditDialog = false
},
async deleteUser(user) {
await axios.delete(`/api/users/${user.id}`)
this.users = this.users.filter(u => u.id !== user.id)
},
cancelEdit() {
this.editingUser = null
this.showEditDialog = false
},
},
}
</script>
```
以上代码仅供参考,具体实现需要根据您的后端接口和数据结构进行调整。
阅读全文
相关推荐

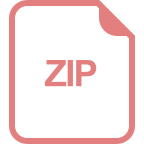
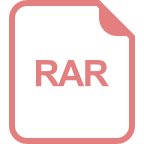
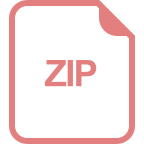
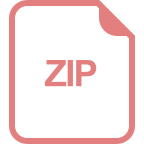
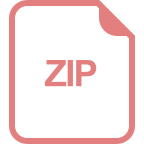
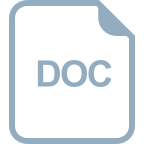
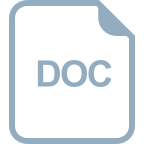
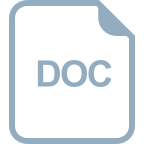
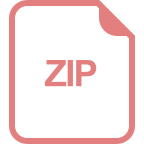
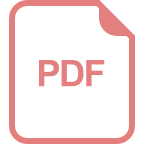
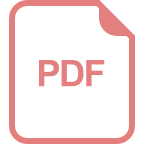
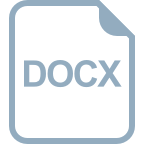