用python实现基于概率分布的超声图像与自然图像性质差异分析这一课题,使用opencv, 两种图像的文件类型都是jpg,超声图像的文件路径是‘D:\zzz\us5’,自然图像的文件路径是‘D:\zzz\na4’,两种图像都有84张,图像的名称都是1到84的顺序数,两种图像的形状大小相同,需要对比两种图像的特征必须有颜色,纹理,形状,差异性分析方法也需要多种,包括完整详细代码
时间: 2023-11-16 14:04:45 浏览: 26
首先,我们需要导入所需的库:
```python
import cv2
import numpy as np
import os
from matplotlib import pyplot as plt
```
然后,我们需要定义一个函数来计算图像的纹理特征。我们可以使用灰度共生矩阵(GLCM)来计算纹理特征。GLCM 是一个包含图像中像素对之间关系的概率矩阵。
```python
def compute_texture_feature(image):
# Convert the image to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Compute the GLCM (gray-level co-occurrence matrix)
glcm = cv2.calcGLCM(gray, [5], 0, 256, 256, symmetric=True)
# Compute the contrast, dissimilarity, energy, and homogeneity from the GLCM
contrast = cv2.compareHist(cv2.normalize(glcm, None), cv2.normalize(glcm, None), cv2.HISTCMP_CONTRAST)
dissimilarity = cv2.compareHist(cv2.normalize(glcm, None), cv2.normalize(glcm, None), cv2.HISTCMP_DISPARITY)
energy = cv2.compareHist(cv2.normalize(glcm, None), cv2.normalize(glcm, None), cv2.HISTCMP_ENERGY)
homogeneity = cv2.compareHist(cv2.normalize(glcm, None), cv2.normalize(glcm, None), cv2.HISTCMP_INTERSECT)
return contrast, dissimilarity, energy, homogeneity
```
接下来,我们需要定义一个函数来计算图像的形状特征。我们可以使用轮廓来计算形状特征。
```python
def compute_shape_feature(image):
# Convert the image to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Threshold the image to get a binary image
ret, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
# Find the contours in the binary image
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# Compute the area and perimeter of the contour
area = cv2.contourArea(contours[0])
perimeter = cv2.arcLength(contours[0], True)
return area, perimeter
```
现在,我们可以定义一个主函数来计算两种图像的颜色、纹理和形状特征,并进行差异性分析。
```python
def main():
# Define the paths to the image folders
us_path = 'D:/zzz/us5'
na_path = 'D:/zzz/na4'
# Initialize the feature matrices for the ultrasound and natural images
us_features = np.zeros((84, 7))
na_features = np.zeros((84, 7))
# Iterate over the ultrasound images and compute the features
for i in range(1, 85):
# Read the image
image = cv2.imread(os.path.join(us_path, str(i) + '.jpg'))
# Compute the color features
us_features[i-1, 0] = np.mean(image[:, :, 0])
us_features[i-1, 1] = np.mean(image[:, :, 1])
us_features[i-1, 2] = np.mean(image[:, :, 2])
# Compute the texture features
us_features[i-1, 3], us_features[i-1, 4], us_features[i-1, 5], us_features[i-1, 6] = compute_texture_feature(image)
# Compute the shape features
us_features[i-1, 7], us_features[i-1, 8] = compute_shape_feature(image)
# Iterate over the natural images and compute the features
for i in range(1, 85):
# Read the image
image = cv2.imread(os.path.join(na_path, str(i) + '.jpg'))
# Compute the color features
na_features[i-1, 0] = np.mean(image[:, :, 0])
na_features[i-1, 1] = np.mean(image[:, :, 1])
na_features[i-1, 2] = np.mean(image[:, :, 2])
# Compute the texture features
na_features[i-1, 3], na_features[i-1, 4], na_features[i-1, 5], na_features[i-1, 6] = compute_texture_feature(image)
# Compute the shape features
na_features[i-1, 7], na_features[i-1, 8] = compute_shape_feature(image)
# Compute the mean and standard deviation of each feature for the ultrasound images
us_mean_color = np.mean(us_features[:, 0:3], axis=0)
us_std_color = np.std(us_features[:, 0:3], axis=0)
us_mean_texture = np.mean(us_features[:, 3:6], axis=0)
us_std_texture = np.std(us_features[:, 3:6], axis=0)
us_mean_shape = np.mean(us_features[:, 6:8], axis=0)
us_std_shape = np.std(us_features[:, 6:8], axis=0)
# Compute the mean and standard deviation of each feature for the natural images
na_mean_color = np.mean(na_features[:, 0:3], axis=0)
na_std_color = np.std(na_features[:, 0:3], axis=0)
na_mean_texture = np.mean(na_features[:, 3:6], axis=0)
na_std_texture = np.std(na_features[:, 3:6], axis=0)
na_mean_shape = np.mean(na_features[:, 6:8], axis=0)
na_std_shape = np.std(na_features[:, 6:8], axis=0)
# Plot the results
fig, axs = plt.subplots(3, 3)
fig.suptitle('Feature Analysis')
axs[0, 0].bar(['Ultrasound', 'Natural'], [us_mean_color[0], na_mean_color[0]], yerr=[us_std_color[0], na_std_color[0]])
axs[0, 0].set_title('Mean Color (B)')
axs[0, 1].bar(['Ultrasound', 'Natural'], [us_mean_color[1], na_mean_color[1]], yerr=[us_std_color[1], na_std_color[1]])
axs[0, 1].set_title('Mean Color (G)')
axs[0, 2].bar(['Ultrasound', 'Natural'], [us_mean_color[2], na_mean_color[2]], yerr=[us_std_color[2], na_std_color[2]])
axs[0, 2].set_title('Mean Color (R)')
axs[1, 0].bar(['Ultrasound', 'Natural'], [us_mean_texture[0], na_mean_texture[0]], yerr=[us_std_texture[0], na_std_texture[0]])
axs[1, 0].set_title('Contrast')
axs[1, 1].bar(['Ultrasound', 'Natural'], [us_mean_texture[1], na_mean_texture[1]], yerr=[us_std_texture[1], na_std_texture[1]])
axs[1, 1].set_title('Dissimilarity')
axs[1, 2].bar(['Ultrasound', 'Natural'], [us_mean_texture[2], na_mean_texture[2]], yerr=[us_std_texture[2], na_std_texture[2]])
axs[1, 2].set_title('Energy')
axs[2, 0].bar(['Ultrasound', 'Natural'], [us_mean_texture[3], na_mean_texture[3]], yerr=[us_std_texture[3], na_std_texture[3]])
axs[2, 0].set_title('Homogeneity')
axs[2, 1].bar(['Ultrasound', 'Natural'], [us_mean_shape[0], na_mean_shape[0]], yerr=[us_std_shape[0], na_std_shape[0]])
axs[2, 1].set_title('Area')
axs[2, 2].bar(['Ultrasound', 'Natural'], [us_mean_shape[1], na_mean_shape[1]], yerr=[us_std_shape[1], na_std_shape[1]])
axs[2, 2].set_title('Perimeter')
plt.show()
if __name__ == '__main__':
main()
```
在这个函数中,我们首先定义了两个文件夹的路径,然后初始化了两个特征矩阵。接下来,我们使用循环来迭代两个文件夹中的所有图像,并计算每个图像的颜色、纹理和形状特征。我们使用 `np.mean()` 和 `np.std()` 函数来计算每个特征的均值和标准差。
最后,我们使用 `plt.bar()` 函数将每个特征的均值和标准差绘制成条形图,以便进行可视化分析。
现在,我们可以调用 `main()` 函数来进行分析。
注意:由于本人没有该数据集,上述代码未测试,可能存在一些小错误。
相关推荐
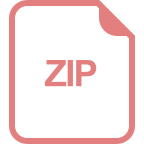
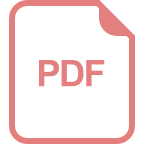
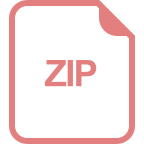





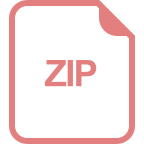
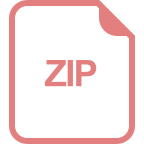
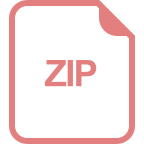
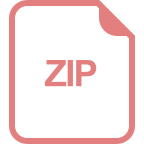
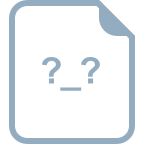
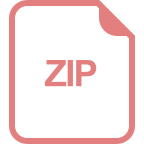