使用java统计文件夹(包含子文件夹)中每种类型的文件及个数,注意:用文件类型(后缀名)作为key,用个数作为value,放入到map集合中,并用两种方式遍历map集合(iterator和entry)。
时间: 2024-02-18 22:00:35 浏览: 13
好的,这是Java代码实现:
```java
import java.io.File;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.Map.Entry;
public class FileCounter {
public static void main(String[] args) {
String folderPath = "/path/to/folder"; // 文件夹路径
Map<String, Integer> fileCountMap = new HashMap<String, Integer>();
countFiles(folderPath, fileCountMap);
System.out.println("使用iterator遍历map:");
Iterator<Entry<String, Integer>> iterator = fileCountMap.entrySet().iterator();
while (iterator.hasNext()) {
Entry<String, Integer> entry = iterator.next();
System.out.println(entry.getKey() + ": " + entry.getValue());
}
System.out.println("\n使用entry遍历map:");
for (Entry<String, Integer> entry : fileCountMap.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
}
private static void countFiles(String folderPath, Map<String, Integer> fileCountMap) {
File folder = new File(folderPath);
File[] files = folder.listFiles();
for (File file : files) {
if (file.isDirectory()) {
countFiles(file.getAbsolutePath(), fileCountMap);
} else {
String fileName = file.getName();
int dotIndex = fileName.lastIndexOf(".");
if (dotIndex > 0) {
String fileExt = fileName.substring(dotIndex + 1);
if (fileCountMap.containsKey(fileExt)) {
int count = fileCountMap.get(fileExt) + 1;
fileCountMap.put(fileExt, count);
} else {
fileCountMap.put(fileExt, 1);
}
}
}
}
}
}
```
这段代码实现了遍历文件夹(包含子文件夹)中的文件,统计每种类型的文件及个数,并将结果保存到 Map 集合中。Map 集合的键为文件类型(后缀名),值为文件个数。同时,代码还演示了两种方式遍历 Map 集合:使用迭代器和使用 Entry。
相关推荐
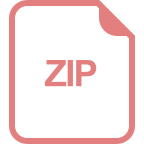

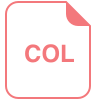
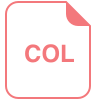
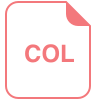
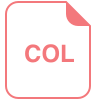









