flask 搭建聊天对话
时间: 2023-10-19 11:42:18 浏览: 43
好的,我可以为你介绍如何使用 Flask 搭建一个简单的聊天对话系统。首先,你需要安装 Flask。你可以在终端中运行以下命令来安装 Flask:
```
pip install flask
```
接下来,你需要创建一个 Flask 应用。你可以创建一个名为 `app.py` 的文件,并在其中添加以下内容:
```python
from flask import Flask, render_template, request
app = Flask(__name__)
@app.route("/")
def home():
return render_template("home.html")
@app.route("/get")
def get_bot_response():
user_text = request.args.get('msg')
# 在这里添加你的 AI 对话逻辑
return "Hello, World!"
if __name__ == "__main__":
app.run()
```
在上面的代码中,我们创建了一个 Flask 应用并定义了两个路由。`home` 路由返回一个包含文本输入框和发送按钮的 HTML 页面。`get_bot_response` 路由获取用户输入的文本并返回一个 AI 对话的响应。你需要在 `get_bot_response` 函数中添加你的 AI 对话逻辑。
接下来,你需要创建一个 HTML 模板文件 `home.html`,内容如下:
```html
<!DOCTYPE html>
<html>
<head>
<title>Chatbot</title>
</head>
<body>
<h1>Chatbot</h1>
<input type="text" id="user-input">
<button onclick="send()">Send</button>
<div id="chat-log"></div>
<script>
function send() {
var userInput = document.getElementById("user-input").value;
var xhr = new XMLHttpRequest();
xhr.open("GET", "/get?msg=" + userInput, true);
xhr.onload = function() {
var botResponse = this.responseText;
var chatLog = document.getElementById("chat-log");
var userRequest = document.createElement("p");
userRequest.innerHTML = userInput;
chatLog.appendChild(userRequest);
var botReply = document.createElement("p");
botReply.innerHTML = botResponse;
chatLog.appendChild(botReply);
};
xhr.send();
}
</script>
</body>
</html>
```
在上面的 HTML 文件中,我们创建了一个文本输入框和发送按钮,并在发送按钮被点击时向 `/get` 路由发送用户输入的文本。在 `/get` 路由响应后,我们将用户输入的文本和 AI 对话的响应添加到一个聊天记录中并显示在页面上。
最后,你可以在终端中运行以下命令启动应用:
```
python app.py
```
现在你可以在浏览器中访问 `http://localhost:5000`,并和你的 AI 进行对话了。
相关推荐
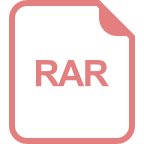















