编制一个能够分析整数、小数、标识符、主要运算符、主要关键字的词法分析器。
时间: 2023-09-28 21:10:13 浏览: 230
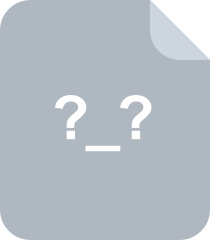
c++ 词法分析器 能够分析出整数、实数,十进制数、八进制数、十六进制数,标识符,主要运算符和主要关键字等

以下是一个简单的词法分析器的实现,可以分析整数、小数、标识符、主要运算符和主要关键字:
```python
import re
# 定义关键字
keywords = {'if', 'else', 'while', 'for', 'int', 'float', 'double', 'char', 'return'}
# 定义符号表
symbol_table = {}
# 正则表达式模式
patterns = {
'int': r'\b\d+\b', # 整数
'float': r'\b\d+\.\d+\b', # 浮点数
'identifier': r'\b[a-zA-Z_]\w*\b', # 标识符
'operator': r'[-+*/%]', # 运算符
'assignment': r'=', # 赋值符号
'delimiter': r'[;,()]' # 分隔符
}
# 词法分析器
def lexer(program):
tokens = []
position = 0
while position < len(program):
match = None
for token_type, pattern in patterns.items():
regex = re.compile(pattern)
match = regex.match(program, position)
if match:
text = match.group(0)
if token_type == 'identifier' and text in keywords:
token_type = text
if token_type == 'identifier':
if text not in symbol_table:
symbol_table[text] = len(symbol_table) + 1
tokens.append((token_type, symbol_table[text]))
else:
tokens.append((token_type, text))
position = match.end(0)
break
if not match:
raise Exception('Illegal character: %s' % program[position])
return tokens
# 测试
program = 'int a = 123; float b = 3.14; while (a < b) { a = a + 1; }'
tokens = lexer(program)
print(tokens)
```
这个词法分析器使用了正则表达式来匹配输入程序中的不同类型的单词。它将每个单词转换为一个元组,其中第一个元素是单词的类型,第二个元素是单词的值。例如,对于输入程序中的 `int a = 123`,词法分析器将返回一个包含三个元组的列表:`[('int', 'int'), ('identifier', 1), ('assignment', '=')]`,其中第二个元组中的值 `1` 表示标识符 `a` 在符号表中的位置。
阅读全文
相关推荐



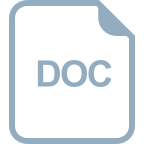
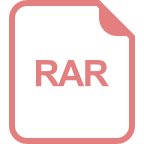
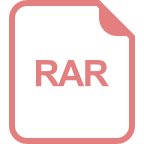
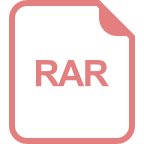
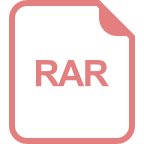
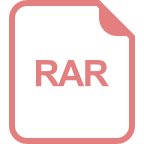
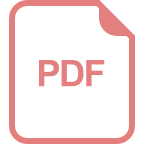
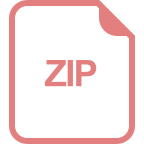
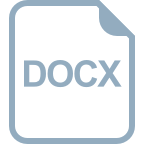
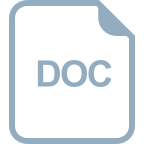
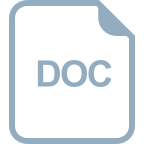
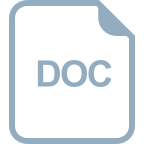
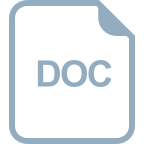
