我需要一份mapreduce编程实例
时间: 2023-08-24 21:06:31 浏览: 206
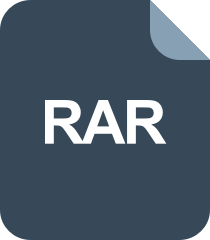
HBase MapReduce完整实例.rar
好的,下面是一个简单的 MapReduce 编程实例:
假设我们有一个文本文件,其中每一行是一个单词。我们想要对这些单词进行计数,并且按照出现次数从大到小进行排序。
首先,我们需要编写 Map 函数,将每个单词映射到一个键值对。键是单词本身,值是 1(表示出现了一次):
```python
def map_function(line):
words = line.strip().split()
for word in words:
yield word, 1
```
然后,我们需要编写 Reduce 函数,将相同单词的计数相加:
```python
def reduce_function(word, counts):
yield word, sum(counts)
```
最后,我们可以使用 Hadoop MapReduce 框架来执行这个程序,其中输入数据是一个文本文件,输出数据是一个按照单词出现次数排序的列表:
```python
from mrjob.job import MRJob
from mrjob.step import MRStep
class WordCount(MRJob):
def steps(self):
return [
MRStep(mapper=self.map_function, reducer=self.reduce_function),
MRStep(reducer=self.reduce_sort)
]
def map_function(self, _, line):
words = line.strip().split()
for word in words:
yield word, 1
def reduce_function(self, word, counts):
yield word, sum(counts)
def reduce_sort(self, word, counts):
yield None, (sum(counts), word)
def reducer_final(self, _, word_counts):
for count, word in sorted(word_counts, reverse=True):
yield word, count
if __name__ == '__main__':
WordCount.run()
```
这个程序使用两个 MapReduce 步骤。第一个步骤是对每个单词计数,第二个步骤是对计数进行排序。
这只是一个简单的例子,实际的 MapReduce 程序可能更加复杂。但是,这个例子可以帮助你理解 MapReduce 的基本思想和编程模型。
阅读全文
相关推荐
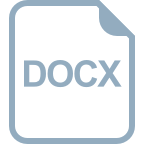
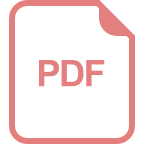















