决策树算法python代码
时间: 2023-11-08 10:57:16 浏览: 97
以下是一个简单的决策树算法的Python代码示例:
```python
import pandas as pd
# 定义数据集
data = {'Outlook': ['Sunny', 'Sunny', 'Overcast', 'Rain', 'Rain', 'Rain', 'Overcast', 'Sunny', 'Sunny', 'Rain', 'Sunny', 'Overcast', 'Overcast', 'Rain'],
'Temperature': ['Hot', 'Hot', 'Hot', 'Mild', 'Cool', 'Cool', 'Cool', 'Mild', 'Cool', 'Mild', 'Mild', 'Mild', 'Hot', 'Mild'],
'Humidity': ['High', 'High', 'High', 'High', 'Normal', 'Normal', 'Normal', 'High', 'Normal', 'Normal', 'Normal', 'High', 'Normal', 'High'],
'Wind': ['Weak', 'Strong', 'Weak', 'Weak', 'Weak', 'Strong', 'Strong', 'Weak', 'Weak', 'Weak', 'Strong', 'Strong', 'Weak', 'Strong'],
'Play': ['No', 'No', 'Yes', 'Yes', 'Yes', 'No', 'Yes', 'No', 'Yes', 'Yes', 'Yes', 'Yes', 'Yes', 'No']}
# 转换为数据框
df = pd.DataFrame(data)
# 定义决策树算法
def decision_tree(data, target_attribute_name, attribute_names, default_class=None):
# 如果数据为空,返回默认类别
if not data: return default_class
# 如果所有目标属性值相同,返回该属性值
elif len(set(data[target_attribute_name])) == 1:
return data[target_attribute_name].iloc[0]
# 如果属性集为空,则返回数据中目标属性值最普遍的值
elif not attribute_names:
return data[target_attribute_name].value_counts().idxmax()
# 否则,按照信息增益最大的属性进行分割
else:
# 计算每个属性的信息增益
gain = {attribute: information_gain(data, attribute, target_attribute_name) for attribute in attribute_names}
# 选择信息增益最大的属性
best_attribute = max(gain, key=gain.get)
# 创建一个新的决策树
tree = {best_attribute: {}}
# 在属性集中删除最佳属性
remaining_attributes = [i for i in attribute_names if i != best_attribute]
# 对于最佳属性的每个值,递归地构建子树
for value in get_values(data, best_attribute):
subtree = decision_tree(
get_examples(data, best_attribute, value),
target_attribute_name,
remaining_attributes,
default_class=get_majority_class(data, target_attribute_name))
# 将新的子树添加到树中
tree[best_attribute][value] = subtree
return tree
# 计算信息熵
def entropy(data, target_attribute_name):
from math import log2
entropy = 0
values = data[target_attribute_name].unique()
for value in values:
fraction = data[target_attribute_name].value_counts()[value] / len(data)
entropy += -fraction * log2(fraction)
return entropy
# 计算信息增益
def information_gain(data, attribute_name, target_attribute_name):
from math import log2
weighted_entropy = 0
values = get_values(data, attribute_name)
for value in values:
subset = get_examples(data, attribute_name, value)
subset_entropy = entropy(subset, target_attribute_name)
fraction = len(subset) / len(data)
weighted_entropy += fraction * subset_entropy
return entropy(data, target_attribute_name) - weighted_entropy
# 获取数据集中某个属性的所有值
def get_values(data, attribute_name):
return set(data[attribute_name])
# 获取数据集中某个属性值的子集
def get_examples(data, attribute_name, value):
return data[data[attribute_name] == value].reset_index(drop=True)
# 获取数据集中目标属性值最普遍的类别
def get_majority_class(data, target_attribute_name):
return data[target_attribute_name].value_counts().idxmax()
# 测试决策树算法
attribute_names = list(df.columns[:-1])
target_attribute_name = df.columns[-1]
tree = decision_tree(df, target_attribute_name, attribute_names)
print(tree)
```
该代码将输出以下决策树:
```
{'Outlook': {'Overcast': 'Yes',
'Rain': {'Wind': {'Strong': 'No', 'Weak': 'Yes'}},
'Sunny': {'Humidity': {'High': 'No', 'Normal': 'Yes'}}}}
```
阅读全文
相关推荐
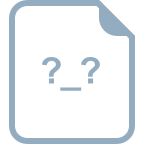
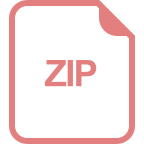
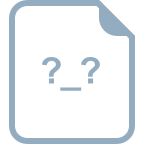
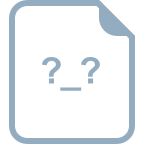
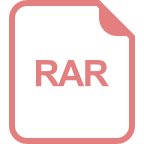
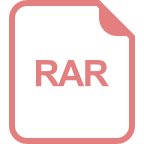
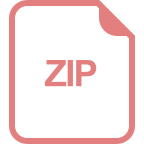
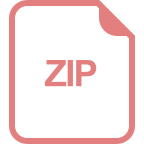
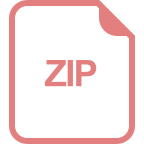
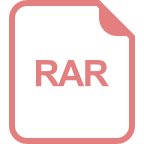
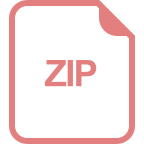

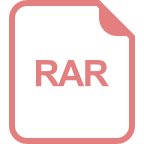
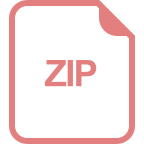
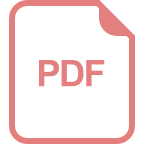
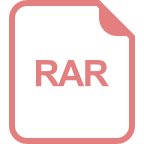
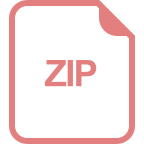
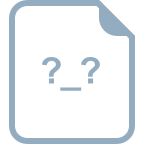