英文单词个数统计python
时间: 2023-07-06 17:38:13 浏览: 130
可以使用Python内置的字符串操作和正则表达式来实现英文单词个数的统计。下面是一个简单的实现:
```python
import re
def count_words(text):
# 将所有非字母字符替换为空格
text = re.sub('[^a-zA-Z]', ' ', text)
# 将连续的空格替换为一个空格
text = re.sub('\s+', ' ', text)
# 分割单词并计数
words = text.split()
return len(words)
```
使用方法:
```python
text = "This is a sample text for counting words."
count = count_words(text)
print(count) # 输出:8
```
这个实现只能统计英文单词,对于其他语言可能会有问题。如果需要支持其他语言,可以使用第三方库,比如 `nltk`。
相关问题
python统计英文单词个数
在Python中,你可以使用内置模块`collections`中的`Counter`类来统计英文单词的数量。以下是一个简单的示例:
```python
from collections import Counter
import re
def count_words(text):
# 将文本转换为小写并删除标点符号
text = re.sub(r'[^\w\s]', '', text.lower())
# 使用split()函数按空格分割成单词列表
words = text.split()
# 使用Counter计算每个单词的频率
word_count = Counter(words)
return word_count
# 测试例子
text = "Hello, world! This is a sample sentence for counting words in Python."
word_counts = count_words(text)
print("Word counts:", word_counts)
输入一个英文字符串 编写程序 统计英文单词个数 python
好的,以下是一个简单的 Python 代码,用于统计英文字符串中单词的个数:
```python
def count_words(s):
"""
统计字符串中单词的个数
"""
s = s.lower() # 转为小写
s = "".join(c if c.isalpha() else " " for c in s) # 只保留字母,其余替换为空格
words = s.split() # 按空格划分单词
return len(words)
s = "This is a test string. It contains some words."
print(count_words(s)) # 输出 8
```
这个函数首先将字符串转为小写,并将所有非字母字符替换为空格。然后按空格划分单词,并统计单词个数。
阅读全文
相关推荐
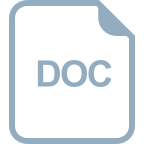








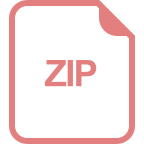


