笔记管理系统c语言
时间: 2023-07-03 10:12:56 浏览: 94
好的,我可以为你提供一些关于笔记管理系统的C语言实现方面的建议。
首先,你需要定义数据结构来存储笔记的内容,可以考虑使用结构体来实现。例如:
```c
struct note {
int id; //笔记ID
char title[50]; //笔记标题
char content[500]; //笔记内容
};
```
然后,你需要实现一些基本的功能,例如添加笔记、删除笔记、修改笔记、查看笔记等。其中,添加笔记可以通过动态内存分配来实现:
```c
struct note *notes = malloc(sizeof(struct note) * MAX_NOTES);
```
这里定义了一个notes数组来存储所有的笔记,MAX_NOTES表示最大笔记数量,可以根据实际需求进行调整。添加笔记的实现可以参考以下代码:
```c
void add_note() {
if (num_notes >= MAX_NOTES) {
printf("笔记数量已达上限!\n");
return;
}
struct note new_note;
printf("请输入笔记标题:");
scanf("%s", new_note.title);
printf("请输入笔记内容:");
scanf("%s", new_note.content);
new_note.id = num_notes + 1;
notes[num_notes] = new_note;
num_notes++;
printf("笔记添加成功!\n");
}
```
其中,num_notes表示当前笔记数量,每次添加笔记时需要先判断笔记数量是否已达上限,如果是,则无法添加。然后,通过scanf函数获取用户输入的笔记标题和内容,并为新笔记分配一个唯一的ID,最后将新笔记加入到notes数组中。
删除笔记的实现可以参考以下代码:
```c
void delete_note() {
int id;
printf("请输入要删除的笔记ID:");
scanf("%d", &id);
int index = find_note_index(id);
if (index == -1) {
printf("未找到该笔记!\n");
return;
}
for (int i = index; i < num_notes - 1; i++) {
notes[i] = notes[i + 1];
}
num_notes--;
printf("笔记删除成功!\n");
}
```
其中,find_note_index是一个辅助函数,用于查找指定ID的笔记在notes数组中的下标。如果未找到,则无法删除。
修改笔记的实现可以参考以下代码:
```c
void edit_note() {
int id;
printf("请输入要修改的笔记ID:");
scanf("%d", &id);
int index = find_note_index(id);
if (index == -1) {
printf("未找到该笔记!\n");
return;
}
struct note *note = ¬es[index];
printf("请输入新的笔记标题:");
scanf("%s", note->title);
printf("请输入新的笔记内容:");
scanf("%s", note->content);
printf("笔记修改成功!\n");
}
```
其中,同样需要先查找指定ID的笔记在notes数组中的下标,然后通过指针修改该笔记的标题和内容。
查看笔记的实现可以参考以下代码:
```c
void view_note() {
int id;
printf("请输入要查看的笔记ID:");
scanf("%d", &id);
int index = find_note_index(id);
if (index == -1) {
printf("未找到该笔记!\n");
return;
}
struct note note = notes[index];
printf("ID:%d\n", note.id);
printf("标题:%s\n", note.title);
printf("内容:%s\n", note.content);
}
```
其中,同样需要先查找指定ID的笔记在notes数组中的下标,然后输出该笔记的详细信息。
以上是笔记管理系统的一些基本实现思路,你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐







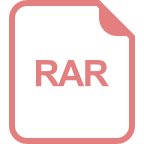



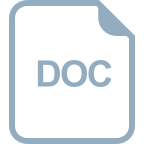



