C++编写任意输入一个字符串统计其中字母a与字母i的个数
时间: 2023-08-27 15:06:49 浏览: 91
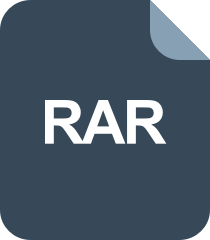
C++统计字符个数
你好啊!关于你的问题,我可以帮你。以下是C语言的代码,可以统计输入字符串中字母a和字母i的个数:
```
#include <stdio.h>
int main() {
char str[100];
int a_count = 0, i_count = 0;
printf("请输入字符串:");
scanf("%s", str);
for(int i = 0; str[i] != '\0'; i++) {
if(str[i] == 'a' || str[i] == 'A') {
a_count++;
} else if(str[i] == 'i' || str[i] == 'I') {
i_count++;
}
}
printf("字符串中字母a的个数是:%d\n", a_count);
printf("字符串中字母i的个数是:%d\n", i_count);
return 0;
}
```
希望这个回答可以帮到你。还有什么其他问题吗?
阅读全文
相关推荐

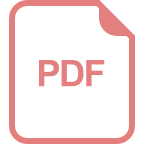
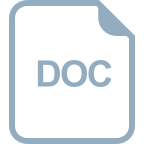
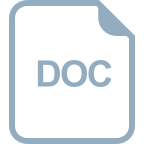

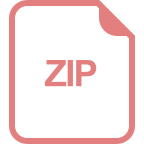
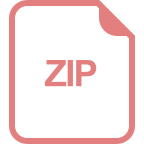
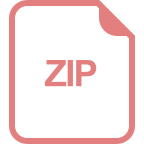
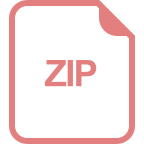