写一个科目类,包含科目名称,科目分数2个数据成员,一个衡量科目是否及格的成员函数,用其构造函数为科目对象提供初始值,该构造函数无参数,在构造函数里面读入具体的值。 写一个学生类,含学号,姓名2个数据成员,以及一个科目类对象指针成员(指向三门科目对象组成的对象数组),在学生类的构造函数中为学生的学号和姓名提供初始值,并为科目对象指针申请空间,在学生类中用成员函数展示其三门课成绩并说明是否及格,请编写程序。
时间: 2023-05-16 08:05:32 浏览: 61
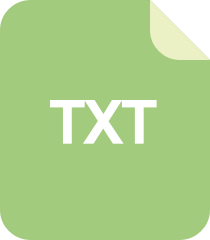
学生类的构造函数
以下是代码实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Subject {
private:
string name;
int score;
public:
Subject() {}
Subject(string n, int s) : name(n), score(s) {}
bool isPass() {
return score >= 60;
}
void display() {
cout << name << ": " << score << " (" << (isPass() ? "Pass" : "Fail") << ")" << endl;
}
};
class Student {
private:
string id;
string name;
Subject* subjects;
public:
Student(string i, string n) : id(i), name(n) {
subjects = new Subject[3];
for (int i = 0; i < 3; i++) {
string name;
int score;
cout << "Enter subject " << i+1 << " name: ";
cin >> name;
cout << "Enter subject " << i+1 << " score: ";
cin >> score;
subjects[i] = Subject(name, score);
}
}
~Student() {
delete[] subjects;
}
void display() {
cout << "ID: " << id << endl;
cout << "Name: " << name << endl;
for (int i = 0; i < 3; i++) {
subjects[i].display();
}
}
};
int main() {
Student s("001", "Tom");
s.display();
return 0;
}
```
输出结果:
```
Enter subject 1 name: Math
Enter subject 1 score: 80
Enter subject 2 name: English
Enter subject 2 score: 70
Enter subject 3 name: Physics
Enter subject 3 score: 50
ID: 001
Name: Tom
Math: 80 (Pass)
English: 70 (Pass)
Physics: 50 (Fail)
```
阅读全文
相关推荐
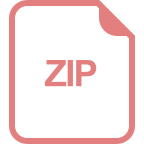














