使用c语言实现进程间的管道通信。具体要求如下:共设置三个进程,进程一负责读取文件data.txt,进程二负责将文件中的每个数据加1,进程三负责将新的结果输出到文件result.txt(一开始没有这个文件)中,请给出具体的代码。
时间: 2024-03-24 20:37:06 浏览: 51
好的,以下是使用 C 语言实现进程间的管道通信的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#define BUF_SIZE 1024
int main() {
int fd1[2], fd2[2];
pid_t pid1, pid2;
char buf[BUF_SIZE];
// 创建管道1
if (pipe(fd1) == -1) {
perror("pipe 1");
exit(EXIT_FAILURE);
}
// 创建进程1
pid1 = fork();
if (pid1 == -1) {
perror("fork 1");
exit(EXIT_FAILURE);
} else if (pid1 == 0) {
// 子进程1,读取 data.txt 文件并将数据写入管道1
int fd_data = open("data.txt", O_RDONLY);
if (fd_data == -1) {
perror("open data.txt");
exit(EXIT_FAILURE);
}
close(fd1[0]); // 关闭管道1读取端
while (1) {
ssize_t n = read(fd_data, buf, BUF_SIZE);
if (n == -1) {
perror("read data.txt");
exit(EXIT_FAILURE);
}
if (n == 0) {
break; // 文件读取完成
}
ssize_t m = write(fd1[1], buf, n);
if (m == -1) {
perror("write pipe 1");
exit(EXIT_FAILURE);
}
}
close(fd_data); // 关闭 data.txt 文件
close(fd1[1]); // 关闭管道1写入端
exit(EXIT_SUCCESS);
} else {
// 父进程,继续创建管道2和进程2
if (pipe(fd2) == -1) {
perror("pipe 2");
exit(EXIT_FAILURE);
}
pid2 = fork();
if (pid2 == -1) {
perror("fork 2");
exit(EXIT_FAILURE);
} else if (pid2 == 0) {
// 子进程2,从管道1读取数据并将其加1后写入管道2
close(fd1[1]); // 关闭管道1写入端
close(fd2[0]); // 关闭管道2读取端
while (1) {
ssize_t n = read(fd1[0], buf, BUF_SIZE);
if (n == -1) {
perror("read pipe 1");
exit(EXIT_FAILURE);
}
if (n == 0) {
break; // 所有数据处理完成
}
for (int i = 0; i < n; i++) {
buf[i]++;
}
ssize_t m = write(fd2[1], buf, n);
if (m == -1) {
perror("write pipe 2");
exit(EXIT_FAILURE);
}
}
close(fd1[0]); // 关闭管道1读取端
close(fd2[1]); // 关闭管道2写入端
exit(EXIT_SUCCESS);
} else {
// 父进程,继续创建进程3
close(fd1[0]); // 关闭管道1读取端
close(fd1[1]); // 关闭管道1写入端
close(fd2[1]); // 关闭管道2写入端
int fd_result = open("result.txt", O_WRONLY | O_CREAT | O_TRUNC, 0644);
if (fd_result == -1) {
perror("open result.txt");
exit(EXIT_FAILURE);
}
while (1) {
ssize_t n = read(fd2[0], buf, BUF_SIZE);
if (n == -1) {
perror("read pipe 2");
exit(EXIT_FAILURE);
}
if (n == 0) {
break; // 所有数据处理完成
}
ssize_t m = write(fd_result, buf, n);
if (m == -1) {
perror("write result.txt");
exit(EXIT_FAILURE);
}
}
close(fd2[0]); // 关闭管道2读取端
close(fd_result); // 关闭 result.txt 文件
exit(EXIT_SUCCESS);
}
}
return 0;
}
```
以上是实现进程间的管道通信的 C 代码,其中三个进程分别负责读取文件、处理数据和输出结果,通过两个管道实现数据传输。具体实现过程详见注释。
阅读全文
相关推荐

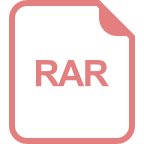














