有没有智能分句的python代码
时间: 2023-07-14 12:12:56 浏览: 123
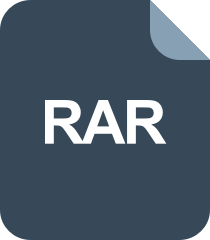
智能分词源代码
是的,Python有许多库可以用于智能分句。其中一个流行的库是NLTK(自然语言工具包),它包含了一个叫做Punkt的模块,可以用于智能分句。下面是一个简单的示例:
```python
import nltk
nltk.download('punkt') # 下载Punkt模块的数据
text = "This is the first sentence. This is the second sentence. And this is the third sentence."
sentences = nltk.sent_tokenize(text)
print(sentences)
```
这将输出以下结果:
```
['This is the first sentence.', 'This is the second sentence.', 'And this is the third sentence.']
```
其中`nltk.sent_tokenize()`函数将输入文本分成句子列表。你可以在这个列表上进行后续处理。
阅读全文
相关推荐
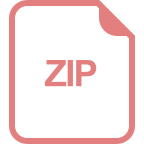
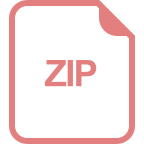
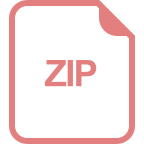
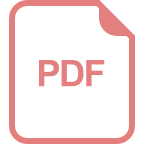
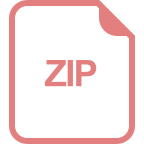
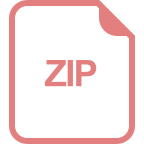
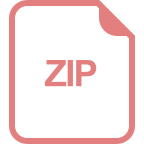
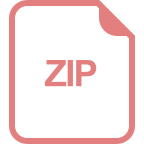
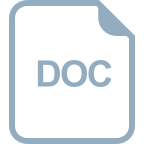
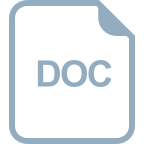
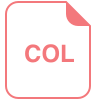
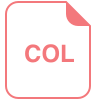
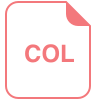
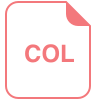
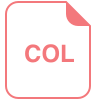
