Given an array of ints, return a new array length 2 containing the first and last elements from the original array. The original array will be length 1 or more.
时间: 2024-02-02 07:04:57 浏览: 190
Sure, here's the solution in Python:
```python
def make_ends(nums):
return [nums[0], nums[-1]]
```
This function takes an input array `nums` and returns a new array containing the first and last elements of the input array. We use the `[0]` index to access the first element of the array, and the `[-1]` index to access the last element of the array.
相关问题
Given 2 int arrays, a and b, each length 3, return a new array length 2 containing their middle elements.
以下是 Python 代码实现:
```python
def middle_way(a, b):
return [a[1], b[1]]
```
这个函数接受两个长度为 3 的整数数组 `a` 和 `b` 作为输入,返回一个新的长度为 2 的数组,其中包含两个输入数组的中间元素。
例如,`middle_way([1, 2, 3], [4, 5, 6])` 的返回值为 `[2, 5]`。
A collection of particles is contained in a linear chamber. They all have the same speed, but some are headed toward the right and others are headed toward the left. These particles can pass through each other without disturbing the motion of the particles, so all the particles will leave the chamber relatively quickly. You will be given the initial conditions by a String init containing at each position an 'L' for a leftward moving particle, an 'R' for a rightward moving particle, or a '.' for an empty location. init shows all the positions in the chamber. Initially, no location in the chamber contains two particles passing through each other. We would like an animation of the process. At each unit of time, we want a string showing occupied locations with an 'X' and unoccupied locations with a '.'. Create a class Animation that contains a method animate that is given an int speed and a String init giving the initial conditions. The speed is the number of positions each particle moves in one time unit. The method will return an array of strings in which each successive element shows the occupied locations at the next time unit. The first element of the return should show the occupied locations at the initial instant (at time = 0) in the 'X', '.' format. The last element in the return should show the empty chamber at the first time that it becomes empty.
以下是Python3代码实现:
```python
class Animation:
def animate(self, speed: int, init: str) -> List[str]:
# 将初始状态转换为列表
particles = list(init)
# 记录每个时间步的状态
result = []
# 当前时间步的状态
current_state = init
while True:
# 记录当前时间步状态
result.append(current_state.replace('R', 'X').replace('L', 'X'))
# 用于记录下一时间步状态的列表
next_state = ['.' for _ in range(len(particles))]
# 标记每个方向的粒子是否已经到达边界
left_reach_edge, right_reach_edge = False, False
# 处理向右移动的粒子
for i in range(len(particles)):
if particles[i] == 'R':
# 粒子向右移动
next_pos = i + speed
# 判断粒子是否到达边界
if next_pos >= len(particles):
right_reach_edge = True
else:
# 判断下一位置是否有粒子
if next_state[next_pos] != 'X':
next_state[next_pos] = 'R'
# 处理向左移动的粒子
for i in range(len(particles) - 1, -1, -1):
if particles[i] == 'L':
# 粒子向左移动
next_pos = i - speed
# 判断粒子是否到达边界
if next_pos < 0:
left_reach_edge = True
else:
# 判断下一位置是否有粒子
if next_state[next_pos] != 'X':
next_state[next_pos] = 'L'
# 更新当前时间步状态
current_state = ''.join(next_state)
# 判断是否所有粒子都已经离开了
if left_reach_edge and right_reach_edge:
result.append(current_state.replace('R', '.').replace('L', '.'))
break
# 更新粒子位置列表
particles = next_state
return result
```
我们定义了一个名为Animation的类,其中包含一个animate方法,用于实现题目所需的功能。在animate方法内部,我们首先将初始状态转换为列表,然后记录每个时间步的状态,并将其存储在result列表中。接下来,我们使用两个for循环分别处理向右移动和向左移动的粒子。对于向右移动的粒子,我们计算它们下一时刻的位置,并将其存储在next_state列表中。如果下一位置没有粒子,则将该粒子标记为向右移动。对于向左移动的粒子,我们采用类似的方法处理。最后,我们更新当前时间步状态并判断是否所有粒子都已经离开了,如果是,我们将最后一个时间步状态添加到结果列表中,并退出循环。最后,我们返回result列表作为最终结果。
阅读全文
相关推荐
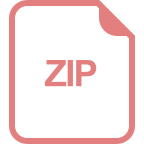
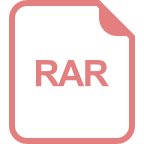
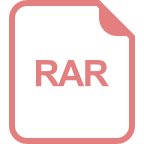











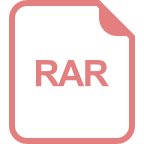
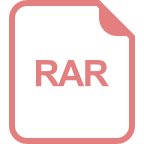
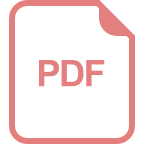