You will be given an array A containing N integers. Find the longest sub-array which contains at most K distinct elements. Input First line contains two integers N, K (1 ≤ K ≤ N ≤ 5 × 105). Next line contains N integers Ai (0 ≤ Ai ≤ 106) - denoting the elements of the array. Output Print two integers L,R (1 ≤ L ≤ R ≤ N) - where L is the left index of the sub-array and R is the right index of the sub-array. If there are multiple sub-arrays with such property, you may print any of them. The elements of the array are numbered from 1 to n from left to right.
时间: 2024-02-25 22:54:24 浏览: 102
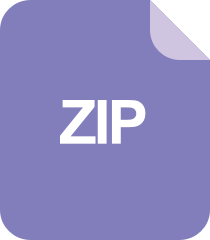
Given-a-string-containing-just-the-characters-and-determine-if-the-inpu
This problem can be solved using a sliding window approach. We will maintain a window of indices [left, right] such that the sub-array A[left, right] contains at most K distinct elements. We will also maintain a hash table to keep track of the count of each element in the current window.
Initially, left = 1, right = 1, and the hash table is empty. We will iterate over the array A from left to right and for each element A[right], we will update the hash table and the number of distinct elements in the current window. If the number of distinct elements is greater than K, we will move the left pointer to the right and update the hash table and the number of distinct elements accordingly. We will continue this process until the number of distinct elements is less than or equal to K.
We will keep track of the maximum length of the sub-array found so far and the corresponding indices. At the end, we will output the indices of the longest sub-array found.
Here's the Python code that implements this approach:
```python
n, k = map(int, input().split())
a = list(map(int, input().split()))
left = right = 0
count = {}
max_len = 0
max_indices = (1, 1)
while right < n:
# Update count of current element
count[a[right]] = count.get(a[right], 0) + 1
# Update number of distinct elements
while len(count) > k:
count[a[left]] -= 1
if count[a[left]] == 0:
del count[a[left]]
left += 1
# Update maximum sub-array length
if right - left + 1 > max_len:
max_len = right - left + 1
max_indices = (left, right+1)
right += 1
print(max_indices[0], max_indices[1])
```
This code has a time complexity of O(n) and a space complexity of O(k).
阅读全文
相关推荐
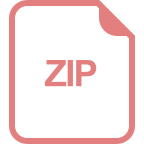
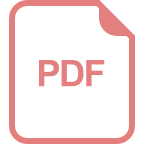














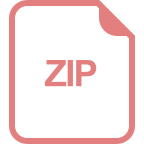
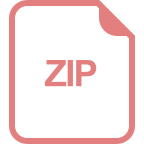