1. PermCheck Check whether array A is a permutation. Task Score 70% Correctness 80% Performance 60% A non-empty array A consisting of N integers is given. A permutation is a sequence containing each element from 1 to N once, and only once. For example, array A such that: A[0] = 4 A[1] = 1 A[2] = 3 A[3] = 2 is a permutation, but array A such that: A[0] = 4 A[1] = 1 A[2] = 3 is not a permutation, because value 2 is missing. The goal is to check whether array A is a permutation. Write a function: class Solution { public int solution(int[] A); } that, given an array A, returns 1 if array A is a permutation and 0 if it is not. For example, given array A such that: A[0] = 4 A[1] = 1 A[2] = 3 A[3] = 2 the function should return 1. Given array A such that: A[0] = 4 A[1] = 1 A[2] = 3 the function should return 0. Write an efficient algorithm for the following assumptions: N is an integer within the range [1..100,000]; each element of array A is an integer within the range [1..1,000,000,000].
时间: 2024-01-21 10:02:32 浏览: 122
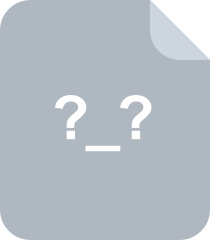
js-leetcode题解之60-permutation-sequence.js
题目大意:
给定一个由N个整数组成的非空数组A,判断A是否为一个排列。排列是一个序列,其中包含1到N的每个元素,每个元素恰好出现一次。
如果是排列,返回1,否则返回0。
解题思路:
题目要求判断一个数组是否为排列,也就是判断数组中是否包含1到N的每个元素,每个元素恰好出现一次。我们可以先对数组进行排序,然后遍历数组,判断当前元素是否等于其下标加1。如果不等于,则说明当前元素缺失或者重复出现,不是排列。
如果遍历完数组都没有发现问题,则说明数组是排列。
时间复杂度为O(NlogN),因为需要对数组进行排序。
Java代码实现:
```
class Solution {
public int solution(int[] A) {
Arrays.sort(A);
for(int i = 0; i < A.length; i++) {
if(A[i] != i + 1) {
return 0;
}
}
return 1;
}
}
```
阅读全文
相关推荐
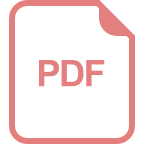













