You are given a positive integer n . In this problem, the MEX of a collection of integers c1,c2,…,ck is defined as the smallest positive integer x which does not occur in the collection c . The primality of an array a1,…,an is defined as the number of pairs (l,r) such that 1≤l≤r≤n and MEX(al,…,ar) is a prime number. Find any permutation of 1,2,…,n with the maximum possible primality among all permutations of 1,2,…,n . Note: A prime number is a number greater than or equal to 2 that is not divisible by any positive integer except 1 and itself. For example, 2,5,13 are prime numbers, but 1 and 6 are not prime numbers. A permutation of 1,2,…,n is an array consisting of n distinct integers from 1 to n in arbitrary order. For example, [2,3,1,5,4] is a permutation, but [1,2,2] is not a permutation (2 appears twice in the array), and [1,3,4] is also not a permutation (n=3 but there is 4 in the array). Input Each test contains multiple test cases. The first line contains the number of test cases t (1≤t≤104 ). The description of the test cases follows. The only line of each test case contains a single integer n (1≤n≤2⋅105 ). It is guaranteed that the sum of n over all test cases does not exceed 2⋅105 . Output For each test case, output n integers: a permutation of 1,2,…,n that achieves the maximum possible primality. If there are multiple solutions, print any of them. 用c++如何实现,并写出题解
时间: 2024-04-11 20:29:10 浏览: 21
以下是一个用C++实现的解题代码:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
// 检查一个数是否为质数
bool isPrime(int num) {
if (num < 2) return false;
for (int i = 2; i * i <= num; i++) {
if (num % i == 0) return false;
}
return true;
}
// 找到最大可能的质数
vector<int> findMaxPrimality(int n) {
vector<int> permutation(n);
for (int i = 0; i < n; i++) {
permutation[i] = i + 1;
}
// 如果n为1或2,则返回原始序列
if (n == 1 || n == 2) return permutation;
// 如果n为偶数,将最后两个数交换位置
if (n % 2 == 0) {
swap(permutation[n-1], permutation[n-2]);
}
// 将质数放在前面,非质数放在后面
for (int i = 2; i <= n; i++) {
if (isPrime(i)) {
swap(permutation[i-1], permutation[n-1]);
break;
}
}
return permutation;
}
int main() {
int t;
cin >> t;
while (t--) {
int n;
cin >> n;
vector<int> permutation = findMaxPrimality(n);
for (int i = 0; i < n; i++) {
cout << permutation[i] << " ";
}
cout << endl;
}
return 0;
}
```
解题思路:
1. 首先,我们考虑特殊情况,当n为1或2时,最大可能的质数就是原始序列。
2. 对于n为偶数的情况,我们可以将最后两个数交换位置,这样可以保证最后一个数不会是质数,因为偶数除2外都不是质数。
3. 对于n为奇数的情况,我们可以将最后一个质数放在序列的最后,其他非质数放在其他位置。我们可以通过遍历从2到n的数字,找到第一个质数,并将其与最后一个数字交换位置。
4. 最后输出得到的排列即为最大可能的质数排列之一。
希望这个解题思路对你有帮助!
相关推荐
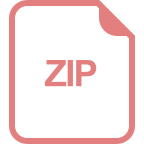
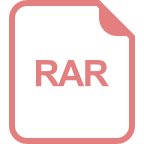
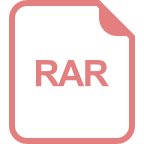












