用python代码实现开心消消乐的小游戏
时间: 2023-07-27 21:24:28 浏览: 120
好的,下面是一个简单的开心消消乐游戏的Python实现,使用了Pygame库:
```python
import pygame
import random
# 初始化游戏
pygame.init()
# 游戏窗口大小
WINDOW_WIDTH = 640
WINDOW_HEIGHT = 480
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
YELLOW = (255, 255, 0)
# 创建游戏窗口
game_window = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
pygame.display.set_caption("开心消消乐")
# 加载游戏图片
ball_images = []
for i in range(1, 6):
ball_images.append(pygame.image.load(f"ball{i}.png"))
# 球的大小和间距
BALL_SIZE = 60
BALL_SPACING = 10
# 生成随机球的颜色
def generate_random_color():
return random.choice([RED, GREEN, BLUE, YELLOW])
# 生成随机球的图片
def generate_random_ball_image():
return random.choice(ball_images)
# 生成随机的球
def generate_random_ball(x, y):
return {
"x": x,
"y": y,
"color": generate_random_color(),
"image": generate_random_ball_image()
}
# 生成随机的球列表
def generate_random_balls(num_balls):
balls = []
for i in range(num_balls):
x = (i % 8) * (BALL_SIZE + BALL_SPACING) + BALL_SPACING
y = (i // 8) * (BALL_SIZE + BALL_SPACING) + BALL_SPACING
balls.append(generate_random_ball(x, y))
return balls
# 绘制球到屏幕
def draw_balls(balls):
for ball in balls:
game_window.blit(ball["image"], (ball["x"], ball["y"]))
# 检查球是否相邻
def is_adjacent(ball1, ball2):
return abs(ball1["x"] - ball2["x"]) <= BALL_SIZE + BALL_SPACING and \
abs(ball1["y"] - ball2["y"]) <= BALL_SIZE + BALL_SPACING
# 寻找相邻的球
def find_adjacent_balls(balls, ball):
adjacent_balls = [ball]
stack = [ball]
while len(stack) > 0:
current_ball = stack.pop()
for other_ball in balls:
if other_ball not in adjacent_balls and is_adjacent(current_ball, other_ball) and other_ball["color"] == ball["color"]:
adjacent_balls.append(other_ball)
stack.append(other_ball)
return adjacent_balls
# 删除相邻的球
def remove_adjacent_balls(balls, adjacent_balls):
for ball in adjacent_balls:
balls.remove(ball)
# 移动球到空缺位置
def move_balls(balls):
for i in range(len(balls)):
if balls[i] is None:
balls[i] = generate_random_ball(i % 8 * (BALL_SIZE + BALL_SPACING) + BALL_SPACING, i // 8 * (BALL_SIZE + BALL_SPACING) + BALL_SPACING)
# 检查游戏是否结束
def is_game_over(balls):
for ball in balls:
if find_adjacent_balls(balls, ball) != [ball]:
return False
return True
# 游戏循环
def game_loop():
num_balls = 64
balls = generate_random_balls(num_balls)
selected_ball = None
game_over = False
while not game_over:
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.MOUSEBUTTONDOWN:
x, y = event.pos
i = y // (BALL_SIZE + BALL_SPACING) * 8 + x // (BALL_SIZE + BALL_SPACING)
if i < num_balls:
ball = balls[i]
if selected_ball is None:
selected_ball = ball
elif selected_ball == ball:
selected_ball = None
elif is_adjacent(selected_ball, ball):
adjacent_balls = find_adjacent_balls(balls, selected_ball)
if len(adjacent_balls) >= 2:
remove_adjacent_balls(balls, adjacent_balls)
move_balls(balls)
selected_ball = None
game_window.fill(WHITE)
draw_balls(balls)
if is_game_over(balls):
font = pygame.font.Font(None, 36)
text = font.render("游戏结束!", True, BLACK)
text_rect = text.get_rect(center=(WINDOW_WIDTH // 2, WINDOW_HEIGHT // 2))
game_window.blit(text, text_rect)
pygame.display.update()
# 退出游戏
pygame.quit()
quit()
# 启动游戏
game_loop()
```
运行上述代码,可以看到一个简单的开心消消乐游戏。玩家可以点击相邻的两个颜色相同的球,将它们消除,直到所有的球都被消除为止。当没有相邻的球可以消除时,游戏结束。
注意:上述代码中的游戏图片 ball1.png 到 ball5.png 需要你自己准备,可以从网络上搜索到类似的图片。
相关推荐
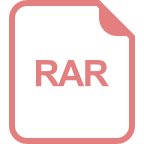
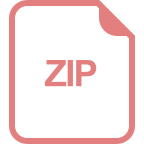
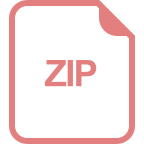














