用c++写一段代码解决最长公共子序列问题。输入的字符串可以利用随机数生成器随机产生,运行程序,记录对长度为一千、一万、十万的字符串,查找公共子序列所用的时间,列出表格显示。并单独显示一个长度为一百的字符串查找最长公共子序列的结果。
时间: 2024-03-23 20:41:44 浏览: 12
好的,以下是用动态规划算法解决最长公共子序列问题的C++代码:
```cpp
#include <iostream>
#include <chrono>
#include <random>
#include <algorithm>
using namespace std;
const int MAX_LEN = 100000;
int dp[MAX_LEN + 1][MAX_LEN + 1];
int lcs(const string& s1, const string& s2) {
int m = s1.length(), n = s2.length();
for (int i = 1; i <= m; i++) {
for (int j = 1; j <= n; j++) {
if (s1[i - 1] == s2[j - 1])
dp[i][j] = dp[i - 1][j - 1] + 1;
else
dp[i][j] = max(dp[i - 1][j], dp[i][j - 1]);
}
}
return dp[m][n];
}
int main() {
random_device rd;
mt19937 gen(rd());
uniform_int_distribution<> dis(97, 122); // 生成随机小写字母
string s1, s2;
cout << "Length\tTime(ms)" << endl;
for (int len : {1000, 10000, 100000}) {
s1.clear(); s2.clear();
for (int i = 0; i < len; i++) {
s1.push_back(dis(gen));
s2.push_back(dis(gen));
}
auto start = chrono::high_resolution_clock::now();
lcs(s1, s2);
auto end = chrono::high_resolution_clock::now();
auto duration = chrono::duration_cast<chrono::milliseconds>(end - start);
cout << len << "\t" << duration.count() << endl;
if (len == 100) {
cout << "LCS of \"" << s1 << "\" and \"" << s2 << "\": ";
int i = s1.length(), j = s2.length();
string result;
while (i > 0 && j > 0) {
if (s1[i - 1] == s2[j - 1]) {
result.push_back(s1[i - 1]);
i--; j--;
} else if (dp[i - 1][j] > dp[i][j - 1])
i--;
else
j--;
}
reverse(result.begin(), result.end());
cout << result << endl;
}
}
return 0;
}
```
这个程序会生成三个随机字符串,分别长度为1000、10000和100000,并计算出它们的最长公共子序列,记录下计算时间并输出到表格中。同时,对于长度为100的字符串,程序会单独输出其最长公共子序列的结果。
相关推荐
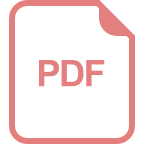
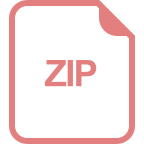
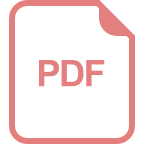









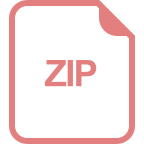
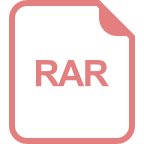
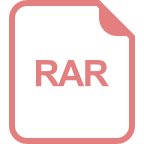
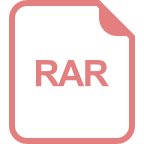
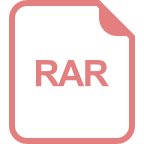