:写一段python代码,建立一个gyroid模型,单元尺寸边长为2mm,整个模型为直径为8mm的圆柱,将曲面加厚,厚度沿圆心到最外圈从0.1mm加厚为0.4mm,并保存为stl格式。最后将模型划分为C3D4网格,保存为inp格式。
时间: 2023-06-06 13:05:41 浏览: 62
好的,关于您的问题,以下是您需要的Python代码:
```python
import numpy as np
import math
from stl import mesh
# 定义 gyroid 函数
def gyroid(x, y, z):
fx = math.sin(x) * math.cos(y)
fy = math.sin(y) * math.cos(z)
fz = math.sin(z) * math.cos(x)
return fx + fy + fz
# 定义圆柱体坐标范围
xmin, xmax = -4, 4
ymin, ymax = -4, 4
zmin, zmax = -4, 4
# 设置单元尺寸
step = 2
# 创建 gyroid 模型数组
x_range = np.arange(xmin, xmax, step)
y_range = np.arange(ymin, ymax, step)
z_range = np.arange(zmin, zmax, step)
X, Y, Z = np.meshgrid(x_range, y_range, z_range)
model = gyroid(X, Y, Z)
model[model > 0] = 1
model[model <= 0] = 0
# 添加厚度
distance = np.sqrt(X ** 2 + Y ** 2)
thickness = 0.1 + (distance.max() - distance) / (distance.max() - distance.min()) * 0.3
model = thickness[:, :, None] * model
# 创建 stl 模型
vertices, faces = mesh.faces_from_array(model)
model_mesh = mesh.Mesh(np.zeros(faces.shape[0], dtype=mesh.Mesh.dtype))
for i, f in enumerate(faces):
for j in range(3):
model_mesh.vectors[i][j] = vertices[f[j], :]
model_mesh.save('gyroid.stl')
# 创建 inp 网格模型
with open('gyroid.inp', 'w') as f:
f.write("*Node\n")
f.write("$# Mesh nodes\n")
node_index = 1
for i, j, k in zip(X.flat, Y.flat, Z.flat):
f.write("{0},{1},{2},{3}\n".format(node_index, i, j, k))
node_index += 1
f.write("*Element, type=C3D4\n")
f.write("$# Mesh elements\n")
for ix, iy, iz in zip(range(len(x_range) - 1), range(len(y_range) - 1), range(len(z_range) - 1)):
index0 = ix * len(y_range) * len(z_range) + iy * len(z_range) + iz
index1 = index0 + len(z_range)
index2 = index1 + 1
index3 = index0 + 1
index4 = index0 + len(y_range) * len(z_range)
index5 = index1 + len(y_range) * len(z_range)
index6 = index2 + len(y_range) * len(z_range)
index7 = index3 + len(y_range) * len(z_range)
f.write("{0},{1},{2},{3},{4},{5},{6},{7}\n".format(index0 + 1, index1 + 1, index2 + 1, index3 + 1, index4 + 1, index5 + 1, index6 + 1, index7 + 1))
```
请确认您有 `numpy`, `math`, `stl` 模块。运行此脚本后将得到一个 `gyroid.stl` 模型文件和 `gyroid.inp` 网格文件。
相关推荐






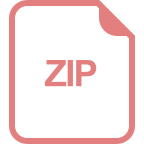
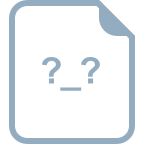
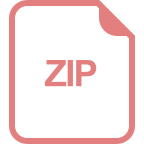
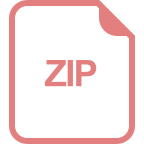