# 遍历输出链表的函数 def print_list(head): p = head while p != -1: print(a[p][0], end=' ') p = a[p][1] print() a = [[2, 2], [5, 0], [1, 3], [7, 4], [3, -1]] pre = p = head = 1 maxpre = maxp = -1 while p != -1: if a[p][0] > a[maxp][0]: maxp = p maxpre = pre pre = p p = a[p][1] # 移动最大值节点到链表开头 补充代码 提示:考虑maxp的可能性有几种,分别该怎么做。 # 遍历输出链表 a print_list(head)
时间: 2024-01-20 22:02:11 浏览: 65
以下是将最大值节点移动到链表开头的代码:
```
# 查找最大值节点
pre = p = head = 1
maxpre = maxp = -1
while p != -1:
if a[p][0] > a[maxp][0]:
maxp = p
maxpre = pre
pre = p
p = a[p][1]
# 移动最大值节点到链表开头
if maxpre != -1:
a[maxpre][1] = a[maxp][1]
a[maxp][1] = head
head = maxp
# 遍历输出链表
print_list(head)
```
当最大值节点在链表中间时,我们需要修改它前面节点的 `a[i][1]` 指针,将其指向最大值节点的后面节点 `a[maxp][1]`。同时,我们需要将最大值节点的 `a[i][1]` 指针指向链表开头,将其作为新的头结点。如果最大值节点已经在链表开头,则不需要进行任何操作。
最后,我们可以调用 `print_list` 函数遍历输出链表。
相关问题
对下面代码每一步含义进行注释 def convert_to_doubly_linked_list(self): if not self.root: return None def convert(root): if not root.left and not root.right: return ListNode(root.val) if not root.left: right_head = convert(root.right) right_tail = right_head while right_tail.next: right_tail = right_tail.next cur_node = ListNode(root.val, None, right_head) right_head.prev = cur_node return cur_node if not root.right: left_tail = convert(root.left) left_head = left_tail while left_head.prev: left_head = left_head.prev cur_node = ListNode(root.val, left_tail, None) left_tail.next = cur_node return cur_node left_tail = convert(root.left) right_head = convert(root.right) left_head = left_tail while left_head.prev: left_head = left_head.prev right_tail = right_head while right_tail.next: right_tail = right_tail.next cur_node = ListNode(root.val, left_tail, right_head) left_tail.next = cur_node right_head.prev = cur_node return left_head return convert(self.root) def inorder_traversal(self, root): if not root: return self.inorder_traversal(root.left) print(root.val, end=' ') self.inorder_traversal(root.right) def print_bst(self): self.inorder_traversal(self.root) print() def traverse_doubly_linked_list(self, head): cur_node = head while cur_node: print(cur_node.val, end=' ') cur_node = cur_node.next print() def reverse_traverse_doubly_linked_list(self, head): cur_node = head while cur_node.next: cur_node = cur_node.next while cur_node: print(cur_node.val, end=' ') cur_node = cur_node.prev print()
这段代码是一个二叉搜索树(BST)转化为双向链表的函数,同时提供了打印BST和遍历双向链表的函数。
- `def convert_to_doubly_linked_list(self):`:定义一个将BST转化为双向链表的函数。
- `if not self.root:`:如果BST为空,则返回None。
- `def convert(root):`:定义一个递归函数,将BST转化为双向链表。
- `if not root.left and not root.right:`:如果该节点没有左右子树,返回一个只包含该节点值的ListNode。
- `if not root.left:`:如果该节点没有左子树,将右子树转化为双向链表,并将节点值作为新的头结点,返回该节点。
- `if not root.right:`:如果该节点没有右子树,将左子树转化为双向链表,并将节点值作为新的尾结点,返回该节点。
- `left_tail = convert(root.left)`:将左子树转化为双向链表,并返回左子树的尾结点。
- `right_head = convert(root.right)`:将右子树转化为双向链表,并返回右子树的头结点。
- `left_head = left_tail`:将左子树的头结点设置为左子树的尾结点。
- `while left_head.prev:`:找到左子树双向链表的头结点。
- `right_tail = right_head`:将右子树的尾结点设置为右子树的头结点。
- `while right_tail.next:`:找到右子树双向链表的尾结点。
- `cur_node = ListNode(root.val, left_tail, right_head)`:创建一个新的节点,值为当前节点值,左指针指向左子树双向链表的尾结点,右指针指向右子树双向链表的头结点。
- `left_tail.next = cur_node`:将左子树双向链表的尾结点的右指针指向新节点。
- `right_head.prev = cur_node`:将右子树双向链表的头结点的左指针指向新节点。
- `return left_head`:返回双向链表的头结点。
- `return convert(self.root)`:调用递归函数convert并返回结果。
- `def inorder_traversal(self, root):`:定义一个中序遍历BST的函数。
- `if not root:`:如果该节点为空,则返回。
- `self.inorder_traversal(root.left)`:递归遍历左子树。
- `print(root.val, end=' ')`:输出当前节点的值。
- `self.inorder_traversal(root.right)`:递归遍历右子树。
- `def print_bst(self):`:定义一个打印BST的函数。
- `self.inorder_traversal(self.root)`:调用中序遍历函数遍历BST。
- `print()`:输出一个空行。
- `def traverse_doubly_linked_list(self, head):`:定义一个遍历双向链表的函数。
- `cur_node = head`:将当前节点指向链表的头结点。
- `while cur_node:`:遍历整个链表,直到当前节点为空。
- `print(cur_node.val, end=' ')`:输出当前节点的值。
- `cur_node = cur_node.next`:将当前节点指向下一个节点。
- `print()`:输出一个空行。
- `def reverse_traverse_doubly_linked_list(self, head):`:定义一个逆序遍历双向链表的函数。
- `cur_node = head`:将当前节点指向链表的头结点。
- `while cur_node.next:`:找到链表的尾结点。
- `cur_node = cur_node.next`:将当前节点指向下一个节点。
- `while cur_node:`:逆序遍历整个链表,直到当前节点为空。
- `print(cur_node.val, end=' ')`:输出当前节点的值。
- `cur_node = cur_node.prev`:将当前节点指向上一个节点。
- `print()`:输出一个空行。
定义二叉搜索树节点类 class TreeNode: def init(self, val): self.val = val self.left = None self.right = None # 插入节点 def insert(root, val): if not root: return TreeNode(val) if val < root.val: root.left = insert(root.left, val) else: root.right = insert(root.right, val) return root # 顺序遍历二叉搜索树 def inorderTraversal(root): res = [] if not root: return res res += inorderTraversal(root.left) res.append(root.val) res += inorderTraversal(root.right) return res # 将二叉搜索树转换为双向循环链表 def treeToDoublyList(root): if not root: return None inorder = inorderTraversal(root) head = cur = TreeNode(None) for val in inorder: cur.right = TreeNode(val) cur.right.left = cur cur = cur.right head.right.left = cur cur.right = head.right return head.right # 打印二叉搜索树 def printTree(root, space): if not root: return space += 5 printTree(root.right, space) print(' ' * space, root.val) printTree(root.left, space) # 打印双向循环链表 def printList(head): if not head: return print(head.val, end=' <--> ') cur = head.right while cur != head and cur.right != head: print(cur.val, end=' <--> ') cur = cur.right if cur.right == head: print(cur.val) cur = cur.right length = 7 * len(values) - 7 print('^' + ' '*length + '^') print('|' + ' '*length + '|') print('\' + '-'*length + '/') print() # 测试 if name == 'main': root = None values = input('请输入要插入的节点值,以空格分隔:').split() for val in values: root = insert(root, val) bstree = root print('Binary Search Tree:') printTree(bstree, 0) dllist = treeToDoublyList(bstree) print('Doubly Linked List:') printList(dllist) print('------------------------menu--------------------------- \n' '|0.escape \n' '|1.input the BSTree elements \n' '|2.traverse the BSTree \n' '|3.print the LinkedList \n' '|4.output all the elements by forward and backward order\n')将menu函数的作用融入代码中
好的,以下是将menu函数融入代码中的版本:
```python
class TreeNode:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
def insert(root, val):
if not root:
return TreeNode(val)
if val < root.val:
root.left = insert(root.left, val)
else:
root.right = insert(root.right, val)
return root
def inorderTraversal(root):
res = []
if not root:
return res
res += inorderTraversal(root.left)
res.append(root.val)
res += inorderTraversal(root.right)
return res
def treeToDoublyList(root):
if not root:
return None
inorder = inorderTraversal(root)
head = cur = TreeNode(None)
for val in inorder:
cur.right = TreeNode(val)
cur.right.left = cur
cur = cur.right
head.right.left = cur
cur.right = head.right
return head.right
def printTree(root, space):
if not root:
return
space += 5
printTree(root.right, space)
print(' ' * space, root.val)
printTree(root.left, space)
def printList(head):
if not head:
return
print(head.val, end=' <--> ')
cur = head.right
while cur != head and cur.right != head:
print(cur.val, end=' <--> ')
cur = cur.right
if cur.right == head:
print(cur.val)
cur = cur.right
def menu():
while True:
choice = input('请输入您的选择:')
if choice == '0':
break
elif choice == '1':
values = input('请输入要插入的节点值,以空格分隔:').split()
for val in values:
root = insert(root, val)
bstree = root
print('Binary Search Tree:')
printTree(bstree, 0)
elif choice == '2':
inorder = inorderTraversal(root)
print('Inorder Traversal:')
print(inorder)
elif choice == '3':
dllist = treeToDoublyList(root)
print('Doubly Linked List:')
printList(dllist)
elif choice == '4':
inorder = inorderTraversal(root)
forward = 'Forward Order: ' + ' <--> '.join(inorder)
backward = 'Backward Order: ' + ' <--> '.join(inorder[::-1])
print(forward)
print(backward)
else:
print('无效的选项,请重新选择!')
if __name__ == '__main__':
root = None
menu()
```
这个版本中,我们将menu函数放在了主函数外面,并且使用了一个while循环,让用户可以在一个菜单中进行多次操作。在用户选择了不同的选项之后,代码会执行相应的函数并输出结果。
阅读全文
相关推荐
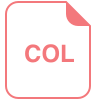













