生成基于pytorch的5层的一维cnn,输入大小为1*8,类别数为3
时间: 2024-02-12 07:07:57 浏览: 21
以下是基于PyTorch实现的5层一维CNN,输入大小为1*8,输出类别数为3的代码:
```python
import torch
import torch.nn as nn
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.conv1 = nn.Sequential(
nn.Conv1d(in_channels=1, out_channels=16, kernel_size=3, stride=1, padding=1),
nn.BatchNorm1d(16),
nn.ReLU(),
nn.MaxPool1d(kernel_size=2, stride=2))
self.conv2 = nn.Sequential(
nn.Conv1d(in_channels=16, out_channels=32, kernel_size=3, stride=1, padding=1),
nn.BatchNorm1d(32),
nn.ReLU(),
nn.MaxPool1d(kernel_size=2, stride=2))
self.conv3 = nn.Sequential(
nn.Conv1d(in_channels=32, out_channels=64, kernel_size=3, stride=1, padding=1),
nn.BatchNorm1d(64),
nn.ReLU(),
nn.MaxPool1d(kernel_size=2, stride=2))
self.conv4 = nn.Sequential(
nn.Conv1d(in_channels=64, out_channels=128, kernel_size=3, stride=1, padding=1),
nn.BatchNorm1d(128),
nn.ReLU(),
nn.MaxPool1d(kernel_size=2, stride=2))
self.conv5 = nn.Sequential(
nn.Conv1d(in_channels=128, out_channels=256, kernel_size=3, stride=1, padding=1),
nn.BatchNorm1d(256),
nn.ReLU(),
nn.MaxPool1d(kernel_size=2, stride=2))
self.fc = nn.Linear(256, 3)
def forward(self, x):
x = self.conv1(x)
x = self.conv2(x)
x = self.conv3(x)
x = self.conv4(x)
x = self.conv5(x)
x = x.view(x.size(0), -1)
x = self.fc(x)
return x
```
在这个网络中,我们使用了5层卷积层,每层都跟着一个BatchNorm层、ReLU激活函数和最大池化层。在网络的输出层,我们使用了一个线性层,输出维度为3(类别数为3)。我们可以通过实例化这个类来创建一个模型:
```python
model = Net()
```
这里我们没有手动指定输入大小,这是因为PyTorch中的卷积层会根据输入的大小自动计算输出的大小。
相关推荐
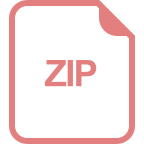
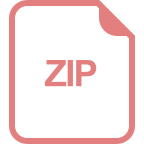














