java判断不同编码格式
时间: 2023-09-30 15:11:40 浏览: 38
在Java中,可以使用以下方法来判断不同的编码格式:
1. 使用Java自带的 Charset 类来判断编码格式:
```java
import java.nio.charset.Charset;
public class CharsetDetector {
public static void main(String[] args) {
byte[] data = "测试".getBytes();
Charset charset = CharsetDetector.detectCharset(data);
System.out.println("Detected charset: " + charset.name());
}
public static Charset detectCharset(byte[] data) {
Charset charset = Charset.forName("UTF-8");
if (data.length >= 2) {
if (data[0] == (byte) 0xff && data[1] == (byte) 0xfe) {
charset = Charset.forName("UTF-16LE");
} else if (data[0] == (byte) 0xfe && data[1] == (byte) 0xff) {
charset = Charset.forName("UTF-16BE");
} else if (data[0] == (byte) 0xef && data[1] == (byte) 0xbb && data[2] == (byte) 0xbf) {
charset = Charset.forName("UTF-8");
} else if (data[0] == (byte) 0x00 && data[1] == (byte) 0x00 && data[2] == (byte) 0xfe && data[3] == (byte) 0xff) {
charset = Charset.forName("UTF-32BE");
} else if (data[0] == (byte) 0xff && data[1] == (byte) 0xfe && data[2] == (byte) 0x00 && data[3] == (byte) 0x00) {
charset = Charset.forName("UTF-32LE");
}
}
return charset;
}
}
```
2. 使用第三方库进行编码检测,如 jchardet:
```java
import org.mozilla.universalchardet.UniversalDetector;
import java.io.*;
public class CharsetDetector {
public static void main(String[] args) throws IOException {
File file = new File("test.txt");
FileInputStream fis = new FileInputStream(file);
byte[] data = new byte[(int) file.length()];
fis.read(data);
fis.close();
UniversalDetector detector = new UniversalDetector(null);
detector.handleData(data, 0, data.length);
detector.dataEnd();
String encoding = detector.getDetectedCharset();
if (encoding != null) {
System.out.println("Detected encoding: " + encoding);
} else {
System.out.println("Unknown encoding");
}
}
}
```
相关推荐
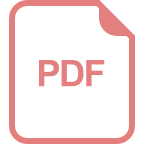
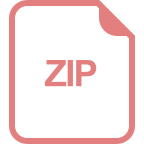
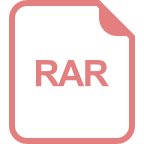














