通过c++使用数据结构完成图书馆图书借阅系统菜单
时间: 2023-10-21 13:07:19 浏览: 114
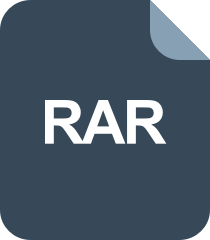
图书借书管理系统 利用c++
好的,下面是一个基于C++的简单图书馆图书借阅系统菜单的代码实现,包括了基于链表的数据结构:
```cpp
#include <iostream>
#include <string>
using namespace std;
struct Book {
string name;
string author;
bool isBorrowed;
Book *next;
};
class Library {
private:
int size;
Book *head;
public:
Library() {
size = 0;
head = NULL;
}
void addBook(string name, string author) {
Book *book = new Book;
book->name = name;
book->author = author;
book->isBorrowed = false;
book->next = head;
head = book;
size++;
cout << "添加图书成功!" << endl;
}
void displayBooks() {
if (size == 0) {
cout << "图书馆里没有任何书籍!" << endl;
} else {
Book *current = head;
cout << "图书馆里有如下书籍:" << endl;
while (current != NULL) {
cout << "书名:" << current->name << endl;
cout << "作者:" << current->author << endl;
cout << "状态:" << (current->isBorrowed ? "已借出" : "未借出") << endl;
cout << endl;
current = current->next;
}
}
}
void borrowBook(string name) {
if (size == 0) {
cout << "图书馆里没有任何书籍!" << endl;
} else {
Book *current = head;
bool foundBook = false;
while (current != NULL) {
if (current->name == name && !current->isBorrowed) {
current->isBorrowed = true;
foundBook = true;
cout << "借书成功!" << endl;
break;
}
current = current->next;
}
if (!foundBook) {
cout << "该书已被借出或不存在!" << endl;
}
}
}
void returnBook(string name) {
if (size == 0) {
cout << "图书馆里没有任何书籍!" << endl;
} else {
Book *current = head;
bool foundBook = false;
while (current != NULL) {
if (current->name == name && current->isBorrowed) {
current->isBorrowed = false;
foundBook = true;
cout << "还书成功!" << endl;
break;
}
current = current->next;
}
if (!foundBook) {
cout << "该书未被借出或不存在!" << endl;
}
}
}
};
int main() {
Library library;
int choice;
string name, author;
do {
cout << "欢迎来到图书馆!请选择您需要的操作:" << endl;
cout << "1. 添加图书" << endl;
cout << "2. 查看图书" << endl;
cout << "3. 借阅图书" << endl;
cout << "4. 归还图书" << endl;
cout << "5. 退出系统" << endl;
cin >> choice;
switch (choice) {
case 1:
cout << "请输入书名和作者名(以空格分隔):" << endl;
cin >> name >> author;
library.addBook(name, author);
break;
case 2:
library.displayBooks();
break;
case 3:
cout << "请输入要借阅的书名:" << endl;
cin >> name;
library.borrowBook(name);
break;
case 4:
cout << "请输入要归还的书名:" << endl;
cin >> name;
library.returnBook(name);
break;
case 5:
cout << "谢谢使用,再见!" << endl;
return 0;
default:
cout << "输入错误,请重新输入!" << endl;
break;
}
} while (true);
return 0;
}
```
该程序实现了一个简单的图书馆图书借阅系统菜单,包括添加图书、查看图书、借阅图书和归还图书等功能。其中,图书信息采用链表的形式存储,每个节点存储一个Book结构体,包括书名、作者名和是否已被借出等信息。用户可以通过菜单选择相应的操作,完成对图书馆的管理。
阅读全文
相关推荐
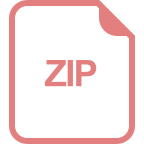
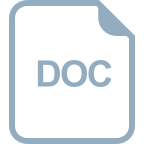
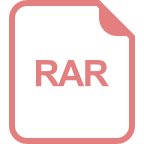
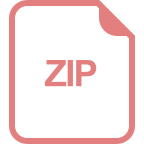
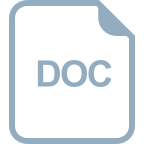
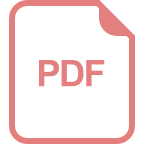
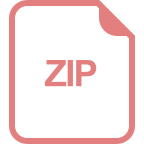
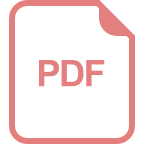
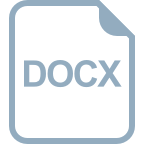
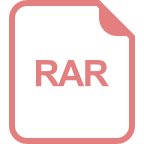
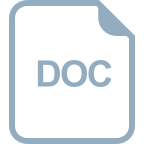
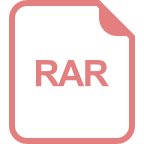
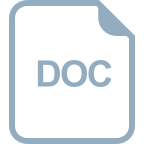
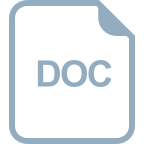
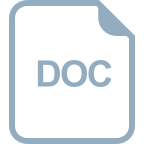
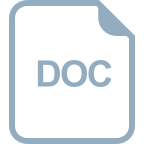
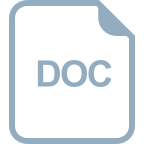