请给我写一个java上传文件到Linux服务器的详细代码
时间: 2024-05-02 09:18:47 浏览: 8
以下是一个简单的Java程序,用于上传文件到Linux服务器:
```
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.InetAddress;
import java.net.Socket;
import java.net.UnknownHostException;
public class UploadFileToLinuxServer {
public static void main(String[] args) {
String serverIpAddress = "192.168.1.100"; // Linux服务器的IP地址
int serverPort = 22; // Linux服务器的SSH端口
String username = "username"; // Linux服务器的用户名
String password = "password"; // Linux服务器的密码
String localFilePath = "/path/to/local/file"; // 本地文件路径
String remoteFilePath = "/path/to/remote/file"; // 远程文件路径
try {
InetAddress serverAddress = InetAddress.getByName(serverIpAddress);
Socket socket = new Socket(serverAddress, serverPort);
InputStream inputStream = socket.getInputStream();
String response = readResponse(inputStream);
System.out.println(response);
String command = "sshpass -p " + password + " ssh " + username + "@" + serverIpAddress + " \"mkdir -p " + remoteFilePath.substring(0, remoteFilePath.lastIndexOf("/")) + "\"";
System.out.println(command);
Process process = Runtime.getRuntime().exec(new String[] { "/bin/bash", "-c", command });
process.waitFor();
command = "scp " + localFilePath + " " + username + "@" + serverIpAddress + ":" + remoteFilePath;
System.out.println(command);
process = Runtime.getRuntime().exec(new String[] { "/bin/bash", "-c", command });
process.waitFor();
response = readResponse(inputStream);
System.out.println(response);
socket.close();
} catch (UnknownHostException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
private static String readResponse(InputStream inputStream) throws IOException {
StringBuilder responseBuilder = new StringBuilder();
byte[] buffer = new byte[1024];
int len;
while ((len = inputStream.read(buffer)) != -1) {
responseBuilder.append(new String(buffer, 0, len));
}
return responseBuilder.toString();
}
}
```
该程序使用了以下的命令来上传文件:
1. `mkdir -p`:创建远程目录,如果目录不存在的话。
2. `scp`:通过SSH协议将本地文件复制到远程服务器上。
该程序使用了`sshpass`命令来自动输入密码,以避免需要手动输入密码。请注意,为了使用`sshpass`命令,需要先在Linux服务器上安装该命令。如果您的Linux服务器没有安装`sshpass`命令,请使用其他方法来上传文件。
另外,请注意,该程序中使用的是SSH协议来上传文件,因此需要确保您的Linux服务器开启了SSH服务。另外,如果您的Linux服务器使用了防火墙,请确保SSH端口是开启的。
相关推荐
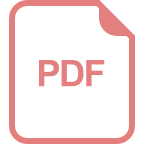














