AES/CBC/PKCS5Padding c#代码实现
时间: 2023-07-07 13:21:09 浏览: 55
以下是C#中使用AES/CBC/PKCS5Padding进行加密和解密的代码示例:
```csharp
using System;
using System.Security.Cryptography;
using System.Text;
public class AesEncryptor
{
private readonly byte[] key;
private readonly byte[] iv;
public AesEncryptor(string key, string iv)
{
this.key = Encoding.UTF8.GetBytes(key);
this.iv = Encoding.UTF8.GetBytes(iv);
}
public string Encrypt(string plainText)
{
byte[] plainBytes = Encoding.UTF8.GetBytes(plainText);
using (Aes aes = Aes.Create())
{
aes.Key = this.key;
aes.IV = this.iv;
aes.Mode = CipherMode.CBC;
aes.Padding = PaddingMode.PKCS7;
ICryptoTransform encryptor = aes.CreateEncryptor();
byte[] encryptedBytes = encryptor.TransformFinalBlock(plainBytes, 0, plainBytes.Length);
return Convert.ToBase64String(encryptedBytes);
}
}
public string Decrypt(string encryptedText)
{
byte[] encryptedBytes = Convert.FromBase64String(encryptedText);
using (Aes aes = Aes.Create())
{
aes.Key = this.key;
aes.IV = this.iv;
aes.Mode = CipherMode.CBC;
aes.Padding = PaddingMode.PKCS7;
ICryptoTransform decryptor = aes.CreateDecryptor();
byte[] decryptedBytes = decryptor.TransformFinalBlock(encryptedBytes, 0, encryptedBytes.Length);
return Encoding.UTF8.GetString(decryptedBytes);
}
}
}
```
使用示例:
```csharp
AesEncryptor encryptor = new AesEncryptor("ThisIsMySecretKey123", "ThisIsMyIv456");
string plainText = "Hello, world!";
string encryptedText = encryptor.Encrypt(plainText);
string decryptedText = encryptor.Decrypt(encryptedText);
Console.WriteLine($"Plain text: {plainText}");
Console.WriteLine($"Encrypted text: {encryptedText}");
Console.WriteLine($"Decrypted text: {decryptedText}");
```
输出结果:
```
Plain text: Hello, world!
Encrypted text: Y5ZIeK7B8c+/z9J5qMg8Ww==
Decrypted text: Hello, world!
```
相关推荐
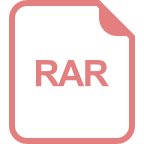
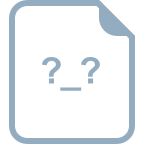














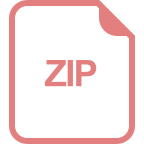