有两个数据集,一个作为x,一个作为y,采用神经网络训练该模型,预测新的测试集,写出代码
时间: 2024-01-21 10:02:15 浏览: 58
好的,下面是使用Python和PyTorch框架编写的神经网络模型代码,用于训练数据集x和y,并对测试集进行预测:
```python
import torch
import torch.nn as nn
import torch.optim as optim
# 定义神经网络模型
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(10, 20) # 输入层10个神经元,隐藏层20个神经元
self.fc2 = nn.Linear(20, 1) # 隐藏层20个神经元,输出层1个神经元
def forward(self, x):
x = torch.sigmoid(self.fc1(x))
x = self.fc2(x)
return x
# 定义训练函数
def train(net, x_train, y_train, epochs, learning_rate):
criterion = nn.MSELoss()
optimizer = optim.SGD(net.parameters(), lr=learning_rate)
for epoch in range(epochs):
optimizer.zero_grad()
outputs = net(x_train)
loss = criterion(outputs, y_train)
loss.backward()
optimizer.step()
# 定义测试函数
def test(net, x_test):
outputs = net(x_test)
return outputs
# 数据集的处理
x_train = torch.randn(100, 10) # 训练数据集x,100个样本,每个样本10个特征
y_train = torch.randn(100, 1) # 训练数据集y,100个样本,每个样本1个标签
x_test = torch.randn(10, 10) # 测试数据集x,10个样本,每个样本10个特征
# 实例化神经网络模型
net = Net()
# 训练神经网络模型
train(net, x_train, y_train, epochs=100, learning_rate=0.01)
# 测试神经网络模型
predictions = test(net, x_test)
print(predictions)
```
以上代码中,我们定义了一个包含一个隐藏层的神经网络模型(输入层10个特征,隐藏层20个神经元,输出层1个标签),使用均方误差(MSE)损失函数进行训练,采用随机梯度下降法(SGD)进行优化。在训练函数中,我们循环训练模型,每个epoch中将模型输出与真实标签计算MSE损失,并根据损失计算梯度并更新模型参数。在测试函数中,我们将测试数据输入到模型中,得到预测结果。最后,我们将测试结果打印输出。
阅读全文
相关推荐
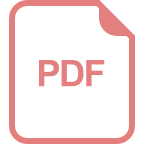















