c++哈希表不用类实现怎么代码实现
时间: 2023-05-25 19:05:11 浏览: 126
哈希表的实现依赖于哈希函数的设计,下面是一个简单的示例,使用数组作为哈希表,不使用类的实现方式:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define TABLE_SIZE 1000
typedef struct entry {
char *key;
int value;
struct entry *next;
} Entry;
Entry *table[TABLE_SIZE] = { NULL };
unsigned int hash(const char *key) {
unsigned int hash = 0;
for (int i = 0; key[i] != '\0'; i++) {
hash = hash * 31 + key[i];
}
return hash % TABLE_SIZE;
}
void put(const char *key, int value) {
Entry *new_entry = (Entry *) malloc(sizeof(Entry));
new_entry->key = strdup(key);
new_entry->value = value;
unsigned int h = hash(key);
new_entry->next = table[h];
table[h] = new_entry;
}
int get(const char *key) {
unsigned int h = hash(key);
Entry *entry = table[h];
while (entry != NULL) {
if (strcmp(entry->key, key) == 0) {
return entry->value;
}
entry = entry->next;
}
return -1;
}
int main() {
put("apple", 1);
put("banana", 2);
put("cherry", 3);
printf("%d\n", get("apple"));
printf("%d\n", get("banana"));
printf("%d\n", get("cherry"));
return 0;
}
```
这里定义了一个`Entry`结构体,表示哈希表的每个元素,其中包含键(`key`)和值(`value`)。哈希表是一个`Entry`指针数组`table`,其中每个元素是一个指向`Entry`结构体的指针。
哈希函数`hash`使用了经典的字符串哈希函数,将字符串转化为一个哈希值,然后使用取模运算得到哈希表的下标。
`put`函数向哈希表中插入一个元素,首先使用`hash`函数计算出该元素的哈希值,然后创建一个新的`Entry`结构体,将键和值赋值给它,并且将该元素插入到哈希表的链表中,链表使用`next`指针实现。
`get`函数用于查找哈希表中的元素,首先根据键值计算出哈希值作为下标,然后遍历该位置的链表,查找键是否匹配,如果找到则返回对应的值。如果没有找到则返回-1。
上述代码仅仅是一个简单的实现,实际情况中还需要考虑冲突解决、扩容、删除等问题。
阅读全文
相关推荐
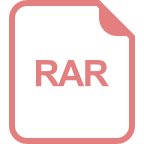
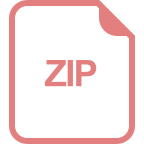
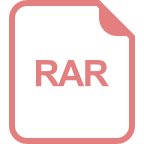
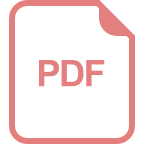
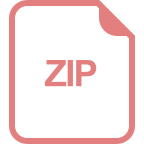
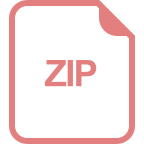
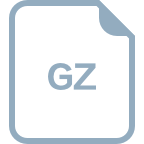
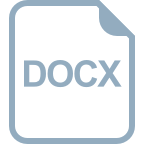
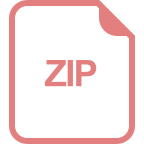
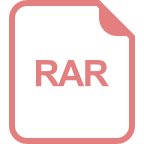
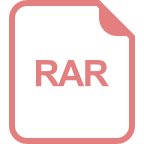
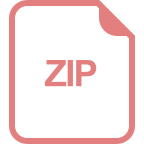
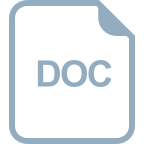
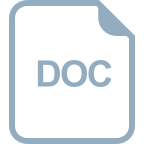
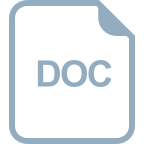